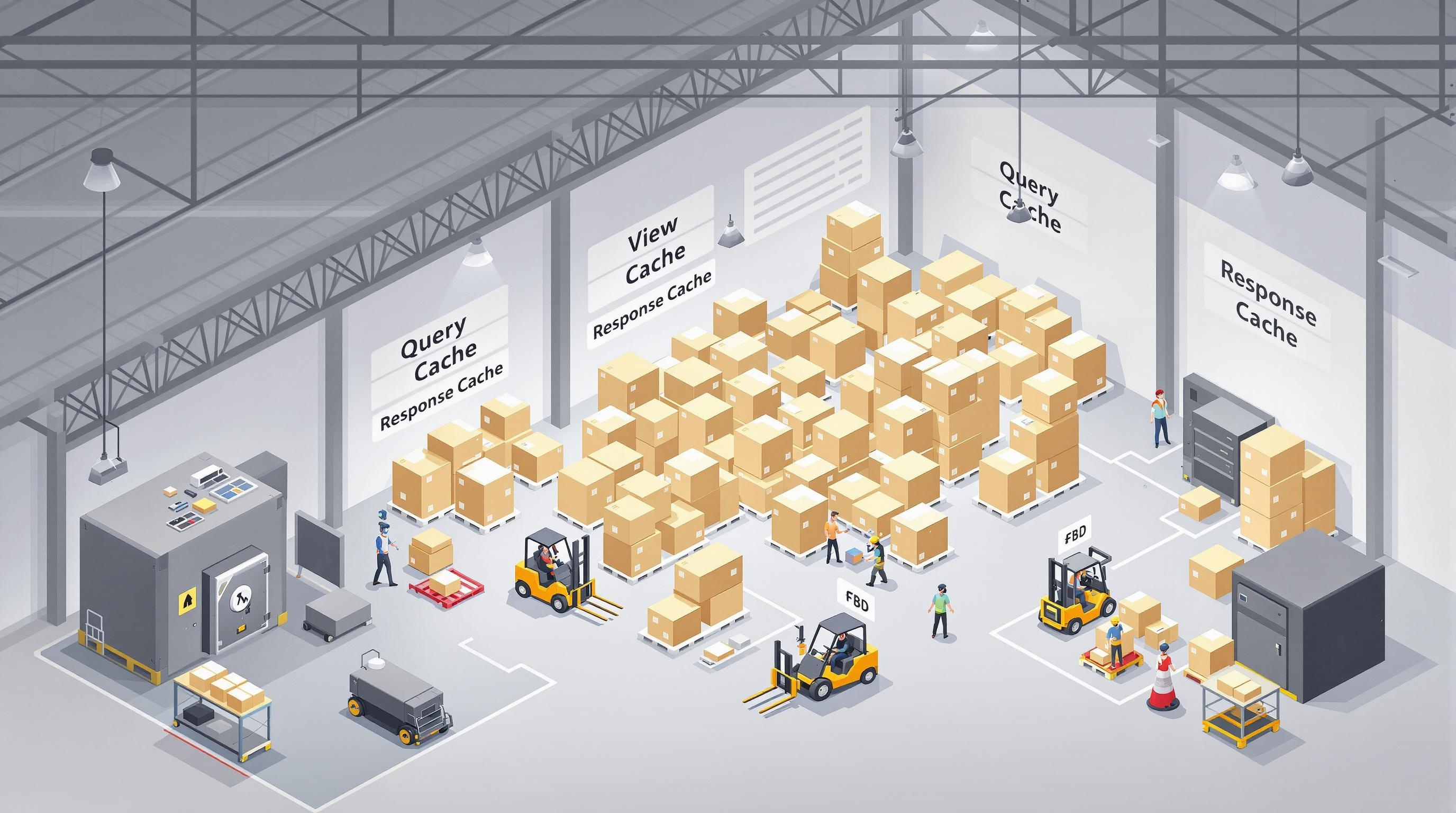
Laravel Caching Strategies: How to Enhance Your App’s Performance
Laravel, one of the most popular PHP frameworks, is known for its elegant syntax and comprehensive feature set. However, as applications grow, performance can become a concern. Implementing effective caching strategies is an essential step to enhancing your Laravel application's performance. In this guide, we'll explore various caching techniques that can help you optimize your Laravel application, ensuring faster load times and a better user experience.
Understanding Caching in Laravel
Caching is the process of storing data in a high-speed data storage layer to reduce the time to retrieve that data. In Laravel, caching can be used for various purposes, such as storing rendered views, database query results, API responses, and more. By leveraging Laravel's robust caching system, developers can significantly reduce the load on their servers and enhance application performance.
Benefits of Caching
- Improved Response Time: Caching reduces the time to access data, resulting in faster response times for users.
- Reduced Server Load: By retrieving cached data, you lessen the demand on your server’s resources, leading to a more efficient infrastructure.
- Better Scalability: Applications that implement caching can handle more requests without compromising performance.
Laravel Caching Tools and Drivers
Laravel provides a simple and unified API for various caching backends, including:
- File Cache: Stores cache data in the file system. It's useful for small applications or local development but may become inefficient at scale.
- Database Cache: Stores cache data in the database, suitable for shared hosting environments but can increase database load.
- Redis Cache: Utilizes Redis, a powerful in-memory data structure store. It's ideal for larger applications requiring quick access.
- Memcached: An in-memory caching system designed for speeding up dynamic web applications by alleviating database load.
Configuring Cache in Laravel
To configure caching in Laravel, you need to set the default cache driver in the config/cache.php file. For example:
'default' => env('CACHE_DRIVER', 'file')
Additionally, you can configure different cache stores and specify their respective configurations.
Effective Caching Strategies
Once you've chosen the appropriate cache drivers for your Laravel application, consider employing these strategies to maximize caching benefits:
1. Query Caching
Laravel's remember method allows easy caching of database queries. For instance:
$users = Cache::remember('users', $seconds, function() { return DB::table('users')->get(); });
This snippet caches the results of a user query for a specified number of seconds, reducing database load on subsequent requests.
2. View Caching
Laravel's route:cache artisan command is used to cache your application's routes, which significantly speeds up route registration:
php artisan route:cache
Be mindful to clear the route cache using php artisan route:clear whenever you make changes to your routes.
3. Response Caching
Response caching stores a full HTTP response, making it easy to return a cached response if the conditions match later:
Route::get('/profile', function() { return Cache::remember('profile-response', $seconds, function() { return response('profile data'); }); });
Advanced Caching Techniques
For high-performance requirements, consider these advanced techniques:
1. Cache Tagging
With Redis or Memcached, Laravel supports cache tagging, allowing you to tag related cache items and manage them together:
Cache::tags(['people', 'artists'])->put('John Doe', $value, $minutes);
This technique is beneficial for clearing groups of cached data without affecting other cached data.
2. Cache Locking
Cache locking can prevent data race conditions by allowing only one process to modify the cache:
$lock = Cache::lock('foo', 10); if ($lock->get()) { // Perform the task... $lock->release(); }
This is particularly useful when handling distributed systems.
Ensuring Cache Security
Caching, while beneficial, can pose security risks if not handled properly.
Considerations for Secure Caching
- Data Sensitivity: Avoid caching sensitive data unless strictly necessary, and ensure it's encrypted.
- Cache Expiration: Define expiration times for cache items to prevent stale data.
- Access Control: Implement access control to restrict who can read or write to the cache.
Conclusion
Implementing effective caching strategies in your Laravel application is vital for maintaining optimal performance, especially as your application scales. By leveraging Laravel's robust caching system and adhering to best practices, developers can ensure their applications are both responsive and resource-efficient.
For more insights into enhancing your Laravel applications, don't forget to explore other resources available on ZapKit. Enhance your development experience with powerful tools and guidance tailored for startup success.
Explore ZapKit's Blogging Tools: Automate content creation and enhance your development journey with ZapKit's comprehensive blogging features. Learn More Now