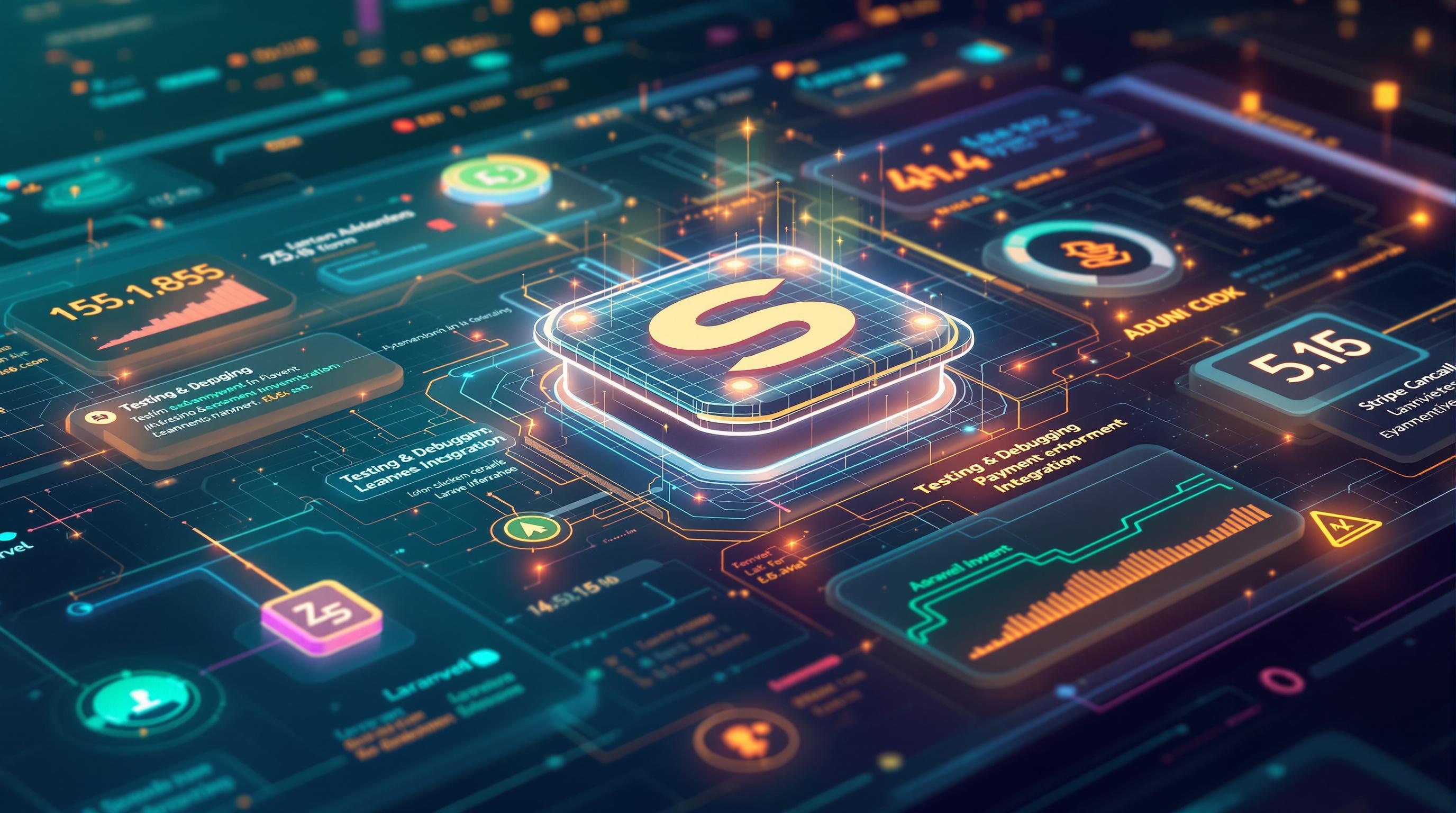
Testing and Debugging Stripe Payments in Laravel Applications
The integration of payment gateways is a critical aspect of modern web applications, particularly for e-commerce businesses. Among the various payment gateways, Stripe stands out for its developer-friendly API and robust functionality. When you're developing applications with Laravel, integrating Stripe is straightforward—but ensuring seamless transactions demands rigorous testing and debugging. In this guide, we will navigate through essential strategies and techniques for effectively testing and debugging Stripe payments within Laravel applications.
Understanding the Basics of Stripe and Laravel Integration
Before diving into testing and debugging, it’s vital to understand the foundational aspects of integrating Stripe with Laravel:
Setting Up Stripe in Laravel
To start with Stripe in a Laravel application, you need to install the Stripe PHP package using Composer:
composer require stripe/stripe-php
After installation, you’ll configure your Stripe API keys. These keys can be found in your Stripe Dashboard under Developers > API keys.
Next, update your environment variables in the .env
file:
STRIPE_KEY=your_stripe_public_key
STRIPE_SECRET=your_stripe_secret_key
Laravel provides services for integrating third-party libraries and enables you to bind these services with application controllers easily.
Basic Laravel Controller for Stripe
Create a new controller using the Artisan command:
php artisan make:controller PaymentController
Within this controller, you can set up actions that handle payment processing, order creation, and any other business logic surrounding your payment workflow:
public function processPayment(Request $request){
\Stripe\Stripe::setApiKey(env('STRIPE_SECRET'));
// Payment process implementation
}
Implementing Testing for Stripe Payments
Testing your Stripe integration involves several practices, utilizing both Laravel's testing features and Stripe's capabilities.
Using Laravel’s Built-in Testing Features
Laravel's testing suite is robust, with features that support both unit and feature tests. To test payment flows effectively:
- Mock Stripe API Requests: Use mocking to simulate Stripe responses. This helps in avoiding real transactions and speeds up the testing process.
- TDD (Test-Driven Development): Write tests before you code actual payment processing logic. This helps ensure your code adheres to desired outcomes.
Stripe’s Testing Toolbox
Stripe provides a wealth of tools that enable you to thoroughly test payment integrations without processing real transactions:
- Test Cards: Stripe has several test card numbers you can use to simulate different scenarios like successful transactions and declines.
- Webhooks: Test your app's webhook integration using
Stripe CLI
that listens to events and sends webhook notifications to your local server.
Debugging Techniques for Laravel and Stripe
When transactions don't go as expected, a series of debugging techniques can be employed:
Check Laravel Logs
Laravel's logging system is an invaluable resource during debugging. Stored in storage/logs/laravel.log
, these logs capture server responses, errors, and exceptions:
Log::info('Stripe response:', [$response]);
Ensure your logging level is set to capture detailed error logs which can be configured in the .env
file:
APP_LOG_LEVEL=debug
Stripe Dashboard Insights
Stripe’s Dashboard offers insights into transactions with logs for each API request made. Check for API logs that display each request and response between your app and Stripe.
Common Errors and Resolutions
Here are some common Stripe-related errors you might encounter along with potential solutions:
- Invalid API Key: Ensure you're using the correct API keys for the environment (test vs. live).
- Card Declined: In testing, simulate specific card decline scenarios using test cards.
Advanced Strategies for Seamless Stripe Payments
Beyond the basics, there are advanced strategies to optimize payment experiences:
Implementing Stripe Elements
Stripe Elements are pre-built UI components for card information that help enhance security and user experience:
// Implementation of Stripe Elements in your frontend
Leverage Stripe Connect for Marketplace Applications
If you’re operating a marketplace, using Stripe Connect is vital for managing multiple sellers and processing payments:
- Set up connected accounts
- Utilize Stripe's API for on-behalf-of charges
Conclusion
Debugging and testing Stripe payments in Laravel applications require a combination of understanding Laravel’s capabilities, leveraging Stripe's robust testing tools, and employing strategic debugging practices. With this guide, start optimizing your Laravel application's payment gateway, ensuring your users experience smooth and secure transactions.
If you're developing your payment systems, consider using ZapKit, a Laravel-powered boilerplate. ZapKit not only provides seamless integration strategies but also aids in automation, potentially turning your startup ideas into reality within hours. Check out ZapKit.dev for more insights and resources that can boost your development process.