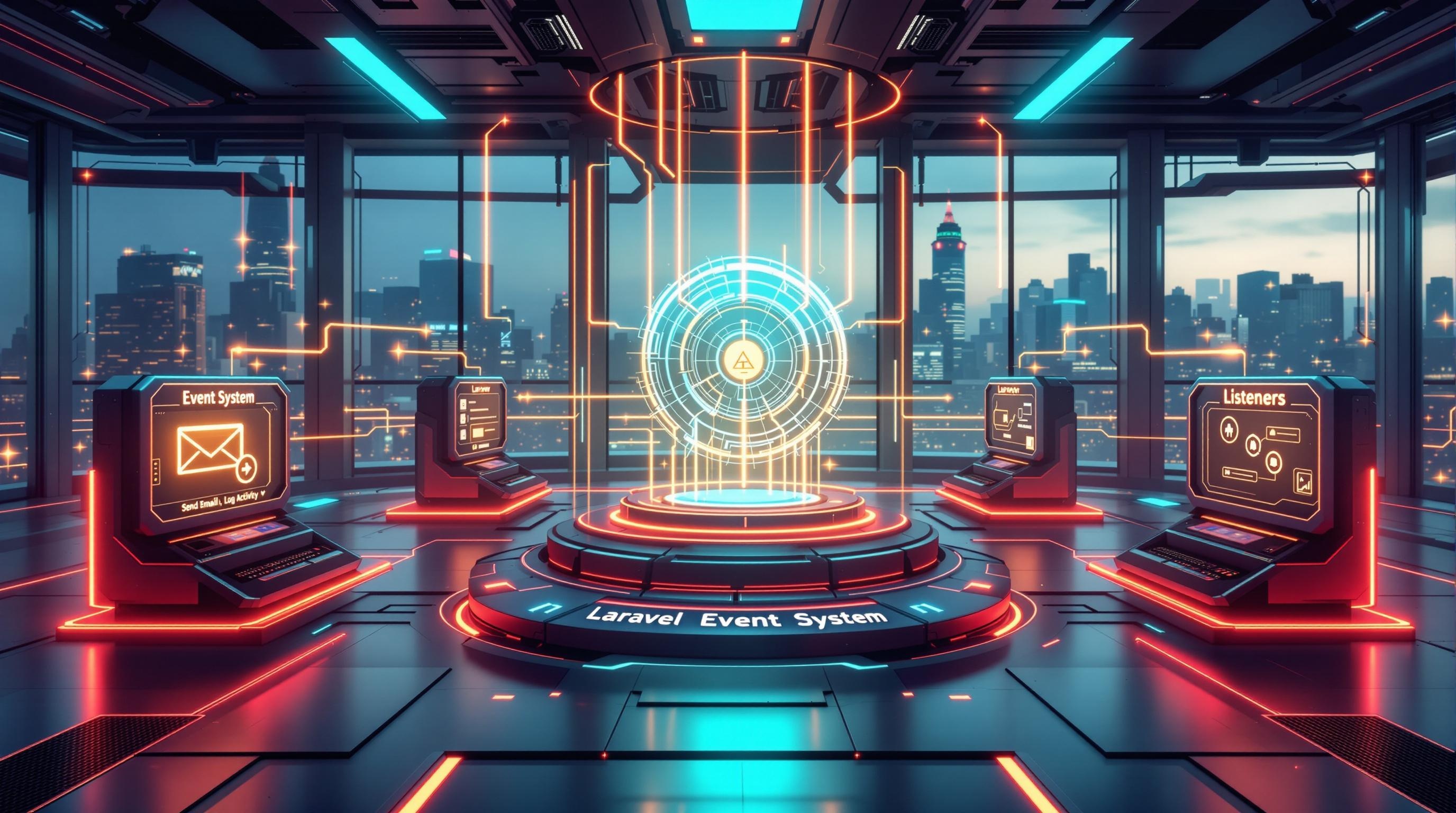
Laravel Events and Listeners: How to Create and Register Them
As a developer working with Laravel, one of the essential skills you can master is efficiently handling events and listeners. Laravel's event system is straightforward and powerful, allowing you to decouple various aspects of your application, maintaining easy readability and flexibility.
Understanding Laravel's Event System
Laravel's event system provides a simple observer pattern implementation, allowing you to subscribe and listen to various events that occur in your application. This modular approach enhances the maintainability and scalability of your project. Think of events as independent, significant actions in your application that other parts may want to respond to. Listeners, in turn, are the responders that perform tasks in response to these events.
Why Use Events and Listeners?
Here are a few reasons why you might want to use events and listeners in your Laravel application:
- Decoupling of logic: You can separate the main logic of your application from additional tasks (like sending emails or logging activities) that can execute independently.
- Scalability: As your application grows, you can add new listeners or modify existing behaviors without altering core functions.
- Reusability: Triggers for specific conditions can be reused across different parts of your application.
How to Create and Register Events and Listeners
The process of creating and registering events and listeners in Laravel is straightforward. In this section, we will dive into a step-by-step guide that simplifies this process.
Step 1: Defining an Event
First, you'll need to define the events that your application will use. Laravel provides a convenient Artisan command for generating an event:
php artisan make:event UserRegistered
This command will create a new event class in the App\Events directory. The event class is where you can define any necessary data properties related to the event and pass them via its constructor.
Step 2: Creating a Listener
Once your event is defined, the next step is to create a listener. Listeners handle the event and perform the specified actions. Use the following command to make a listener:
php artisan make:listener SendWelcomeEmail
This will generate a new listener class in the App\Listeners directory. Within the listener's handle method, you will define the logic to execute when the event is triggered.
Step 3: Registering the Event and Listener
The last step is to register your events and associated listeners within your application. Open the EventServiceProvider file located in the app/Providers directory. Here, you'll list your events and listeners:
protected $listen = [ 'App\Events\UserRegistered' => [ 'App\Listeners\SendWelcomeEmail', ], ];
After defining them within the $listen array, the events and listeners are ready for use.
Triggering Events
Once the event is defined, registered, and listened to, you can trigger it from anywhere in your application. You can call it from a controller, service, or any other place that requires the event to be fired. Here's how you might trigger the UserRegistered event:
event(new UserRegistered($user));
The event function dispatches the event object, which then notifies all registered listeners associated with it.
Managing Events in Laravel
Laravel also provides several artisan commands and tools to manage and work with events more efficiently. For larger applications, consider utilizing Laravel's additional capabilities such as queued listeners, which allow you to handle the processing of events in the background:
Queued Listeners
If you have long-running operations in your listeners, you can queue them to improve performance and user experience. Simply implement the ShouldQueue interface in your listener class:
class SendWelcomeEmail implements ShouldQueue
Don't forget to configure your queue settings appropriately, ensuring your background jobs run smoothly.
Event Subscriptions
Laravel also supports event subscribers, which are classes that can subscribe to multiple events from within the same class. Subscribers should define a subscribe method, which registers event listeners:
public function subscribe(Dispatcher $events) { $events->listen( 'App\Events\UserRegistered', 'App\Listeners\UserEventListener@onUserRegistered' ); $events->listen( 'App\Events\UserLogin', 'App\Listeners\UserEventListener@onUserLogin' ); }
Subscribers are a neat way to group event-driven tasks within a single class, promoting better organization within your project.
Best Practices for Using Events in Laravel
To make the best use of Laravel's events and listeners, keep these practices in mind:
- Keep Listeners Focused: Each listener should handle only one job to keep it simple and maintainable.
- Use Queues Wisely: For operations that might take longer, always consider queuing them.
- Avoid Business Logic in Listeners: Keep the listeners free from complex logic, and delegate tasks to other services or models if needed.
Conclusion
Leveraging the event system in Laravel can significantly enhance the capabilities and robustness of your applications. By creating well-defined events and listeners, you can effectively respond to changes and actions within your application while maintaining a clean and manageable codebase. Whether you are straightforwardly firing an event or employing queued listeners for background job processing, embracing Laravel's event-driven architecture will lead to more efficient and scalable applications.
If you want to dive deeper into how Laravel can accelerate your development process, be sure to explore ZapKit, a Laravel-boilerplate designed to streamline startup creation for developers.