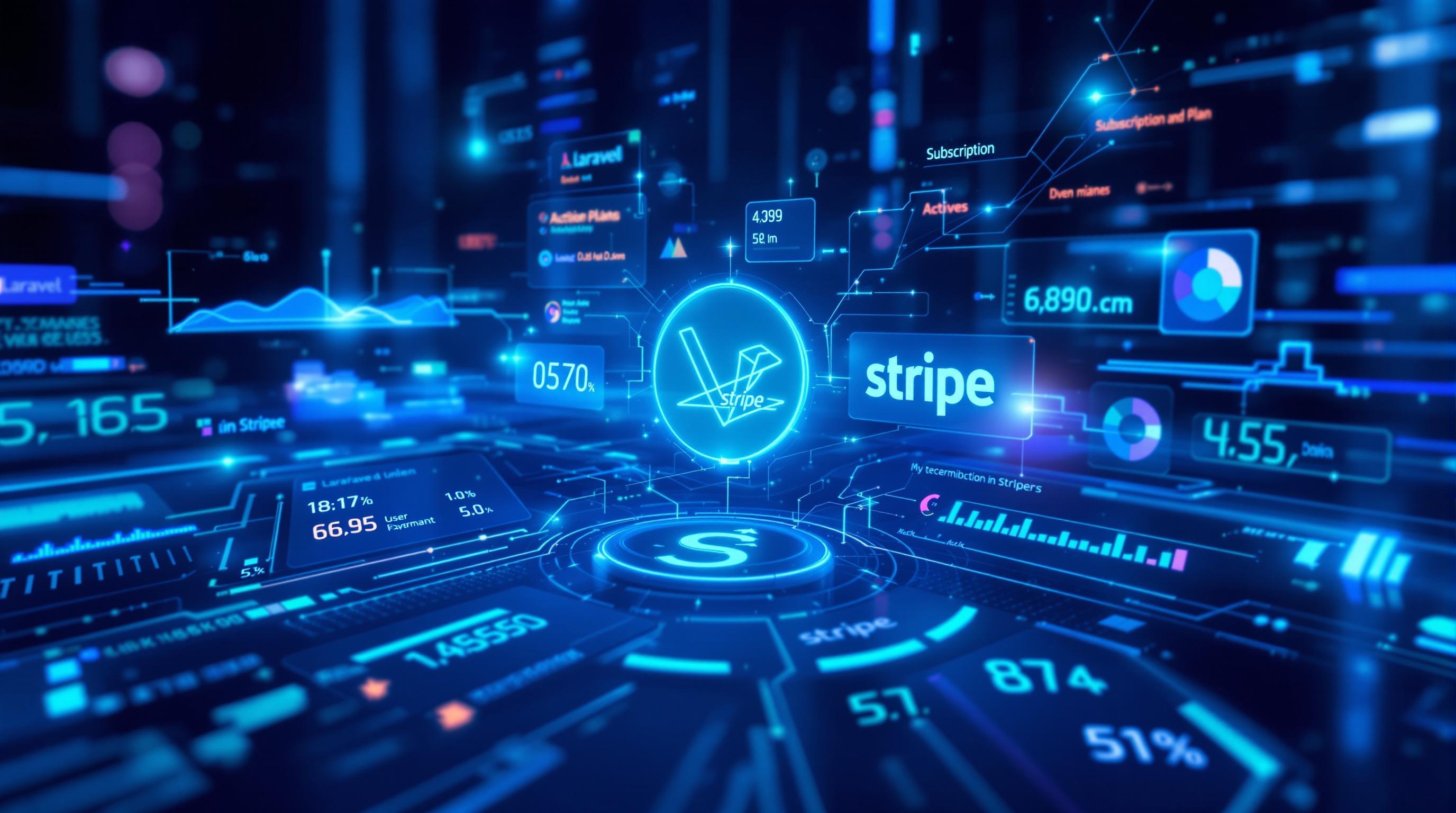
Simplifying Subscription Management in Laravel with Stripe
Managing subscriptions and recurring payments efficiently is pivotal for any business that relies on a subscription model. By harnessing the power of Stripe and Laravel, you can streamline this process and deliver a seamless experience to your users. In this detailed guide, we'll explore how to manage subscriptions in Laravel using Stripe and discuss the benefits of integrating this powerful duo.
Why Choose Stripe for Subscription Management?
Stripe is renowned for its simplicity, comprehensiveness, and reliability. It supports businesses of all sizes by offering:
- Customizable Plans: Create and manage different subscription plans easily.
- Secure Transactions: Benefit from robust security features including fraud prevention.
- Global Reach: Accept payments from customers around the world with multi-currency support.
Setting Up Stripe in Your Laravel Project
Before diving into coding, ensure you have a functional Laravel project. If not, set one up using Composer:
composer create-project --prefer-dist laravel/laravel subscription-app
Step 1: Install Stripe Package
You'll need the Stripe PHP SDK and optionally, Laravel Cashier, which provides an expressive interface for subscription billing with Stripe:
composer require stripe/stripe-php
composer require laravel/cashier
Step 2: Configure Stripe
Set up your environment variables in the .env
file:
STRIPE_KEY=your_stripe_public_key
STRIPE_SECRET=your_stripe_secret_key
Replace your_stripe_public_key
and your_stripe_secret_key
with keys from your Stripe dashboard.
Creating Subscription Models and Plans
Stripe allows you to define various subscription plans that suit your business needs. Once your plans are set up in Stripe, implement the following in Laravel:
Step 1: Create a Subscription Model
Add a new model for subscriptions if not using default models:
php artisan make:model Subscription -m
Adjust the migration to include necessary fields like stripe_id, plan_id
, etc.
Step 2: Plan Controller and Routes
Design a controller to handle subscription requests:
php artisan make:controller SubscriptionController
Set up routes in web.php
or api.php
for managing plans and subscriptions.
Handling Webhooks and Events
Stripe webhooks are critical for managing events like subscription renewals, payments, and cancellations. Configure webhooks in your Stripe dashboard and create a controller in Laravel to handle these:
php artisan make:controller WebhookController
Inside this controller, implement logic for handling different Stripe events:
public function handleWebhook(Request $request) {
// Handle the particular event type
}
Benefits of Using Laravel Cashier
For developers using Laravel, Cashier significantly simplifies the Stripe integration by providing out-of-the-box solutions for subscription management.
- Automated Invoicing: Cashier handles generating billing invoices.
- Flexible Discounts and Coupons: Easily apply discounts to subscription plans.
- Simplified Plan Changes: Cashier makes it straightforward to handle upgrades and downgrades of plans.
- Trial Period Support: Offer trial periods without extensive backend configuration.
Troubleshooting Common Issues
Handling Failed Payments
Failed payments can detrimentally affect user experience. Implement logic to deal with these by integrating dunning solutions or notifying users to update their payment information.
Webhook Configuration Errors
Ensure webhook URL is correctly set in the Stripe dashboard and accessible publicly. This ensures events are duly captured and processed in your Laravel application.
Testing Your Stripe Integration
Before going live, thoroughly test your Stripe integration using the Stripe test mode. Make use of these test credentials and ensure all potential user flows are smoothly handled.
Simulating Events
Use the Stripe CLI to trigger events like payment success, failure, and others to ensure your webhook handling is robust.
Conclusion
With Stripe and Laravel, managing subscriptions becomes accessible and efficient, empowering your business to focus on growth and customer satisfaction. By following best practices and making full use of Laravel Cashier, you can maintain a scalable subscription system with minimal effort. Whether you are a startup looking to launch quickly or an established enterprise, integrating Stripe with Laravel equips you with the tools necessary for effortless subscription management.
Ready to enhance your subscription model with Stripe and Laravel? Explore more about ZapKit to supercharge your development process.