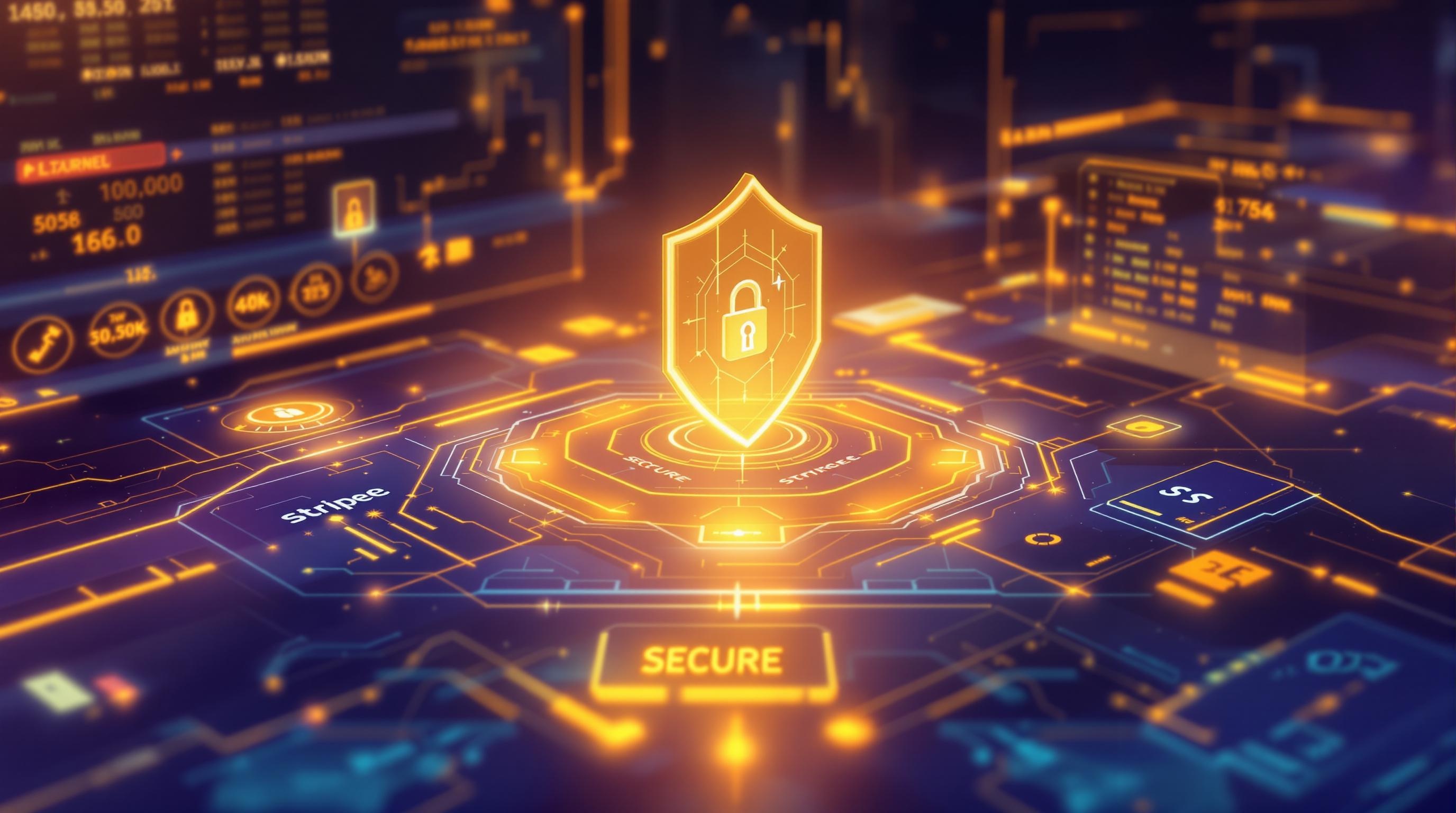
Secure Payment Processing in Laravel Using Stripe APIs
In the rapidly evolving landscape of e-commerce and digital services, processing payments securely and efficiently has become paramount for any online business. Laravel, a popular PHP framework, offers a robust foundation for developing web applications, while Stripe stands out as a leading platform for payment processing due to its powerful APIs and extensive documentation. In this comprehensive guide, we'll explore how to integrate Stripe APIs into a Laravel application to handle payments securely.
Why Choose Stripe for Payment Processing?
Stripe is designed to manage the complexity of payment systems with ease and security. Here are some reasons to choose Stripe for your Laravel application:
- Security: Stripe is PCI DSS compliant, ensuring that your transactions are secure.
- Ease of Integration: Stripe provides a comprehensive suite of APIs that seamlessly integrate with Laravel.
- Scalability: Whether you’re a startup or an enterprise, Stripe can handle your payment processing needs as you grow.
- Global Reach: With support for hundreds of currencies and various payment methods, Stripe allows you to sell anywhere.
Setting Up Your Laravel Application
To begin using Stripe with Laravel, ensure you have a working Laravel application. If you’re starting fresh, you can create a new Laravel project using Composer:
composer create-project --prefer-dist laravel/laravel your-project-name
Once you have your Laravel application set up, you can proceed to install and configure Stripe.
Installing Stripe in Laravel
Stripe provides an official PHP package that you can seamlessly integrate into your Laravel application. To install it, you can use Composer:
composer require stripe/stripe-php
Next, you’ll want to establish your Stripe configuration. Start by adding your Stripe API keys to your .env
file:
STRIPE_KEY=your_stripe_key
STRIPE_SECRET=your_stripe_secret
Make sure to add your actual API keys from your Stripe dashboard. With these keys, your Laravel application can authenticate requests to the Stripe API.
Creating Payment Logic
With Stripe installed, it’s time to create logic for processing payments. Here's a step-by-step guide:
Step 1: Setting Up Routes
Create routes in your web.php
file for handling payment operations:
Route::get('/checkout', 'CheckoutController@index');
Route::post('/charge', 'CheckoutController@charge');
Step 2: Building the Checkout Controller
Create a CheckoutController
to manage the checkout process:
php artisan make:controller CheckoutController
Within the controller, implement the index method to handle the checkout page and the charge method to process payments:
public function charge(Request $request)
{
\Stripe\Stripe::setApiKey(env('STRIPE_SECRET'));
$charge = \Stripe\Charge::create([
'amount' => 9999,
'currency' => 'usd',
'source' => $request->stripeToken,
'description' => 'Test Charge',
]);
return back();
}
Step 3: Creating a View for Checkout
Create a Blade view file for your checkout page, where customers can enter their payment details and complete the transaction. Here's a simple form to get started:
<form action="{{ url('/charge') }}" method="post" id="payment-form">
{{ csrf_field() }}
<div class="form-row">
<label for="card-element">
Credit or debit card
</label>
<div id="card-element"></div>
<div id="card-errors" role="alert"></div>
</div>
<button>Submit Payment</button>
</form>
Use Stripe.js to handle the creation of payment tokens:
<script src="https://js.stripe.com/v3/"></script>
<script>
var stripe = Stripe('{{ env('STRIPE_KEY') }}');
var elements = stripe.elements();
var card = elements.create('card');
card.mount('#card-element');
card.addEventListener('change', function(event) {
var displayError = document.getElementById('card-errors');
if (event.error) {
displayError.textContent = event.error.message;
} else {
displayError.textContent = '';
}
});
var form = document.getElementById('payment-form');
form.addEventListener('submit', function(event) {
event.preventDefault();
stripe.createToken(card).then(function(result) {
if (result.error) {
var errorElement = document.getElementById('card-errors');
errorElement.textContent = result.error.message;
} else {
stripeTokenHandler(result.token);
}
});
});
function stripeTokenHandler(token) {
var form = document.getElementById('payment-form');
var hiddenInput = document.createElement('input');
hiddenInput.setAttribute('type', 'hidden');
hiddenInput.setAttribute('name', 'stripeToken');
hiddenInput.setAttribute('value', token.id);
form.appendChild(hiddenInput);
form.submit();
}
</script>
Testing Your Payment Processing
Before going live, ensure your integration is working correctly:
- Use Stripe's test mode by using the test API keys and pre-defined testing cards.
- Validate the entire payment flow, ensuring that successful payments are captured and invalid payments are handled gracefully.
Best Practices for Secure Payment Processing
Security is critical in payment processing. Here are some best practices:
- Use HTTPS: Always secure your application with HTTPS to encrypt data.
- Environment Variables: Keep your API keys secure by storing them in non-public configuration files like
.env
. - Regular Updates: Keep Stripe libraries and Laravel updated to the latest versions to leverage security patches.
- Validation: Validate all payment-related data to avoid processing incorrect or malicious information.
Advanced Features and Customizations
For businesses looking to expand their payment capabilities, Stripe offers additional features and APIs:
- Recurring Payments: Utilize Stripe's subscription APIs to handle recurring billing.
- Custom Payment Flows: Customize your checkout flow using Stripe Elements and APIs to fit your brand.
- Fraud Protection: Implement Stripe Radar and machine learning tools to detect and prevent fraudulent activities.
Each of these features requires additional setup and consideration of business needs, but greatly enhances your payment processing strategy.
Conclusion
Implementing a secure payment processing system using Stripe APIs within a Laravel application is straightforward, scalable, and effective. By leveraging Laravel’s framework and Stripe’s robust APIs, businesses can create flexible, secure, and reliable payment solutions adaptable to their growth and changing needs. As you integrate Stripe into your application, remember to follow security best practices and continually test your payment processes to maintain the integrity and trustworthiness of your transactions.
Ready to enhance your web application's payment capabilities? Explore more about ZapKit and develop smarter, faster, and safer with Laravel.