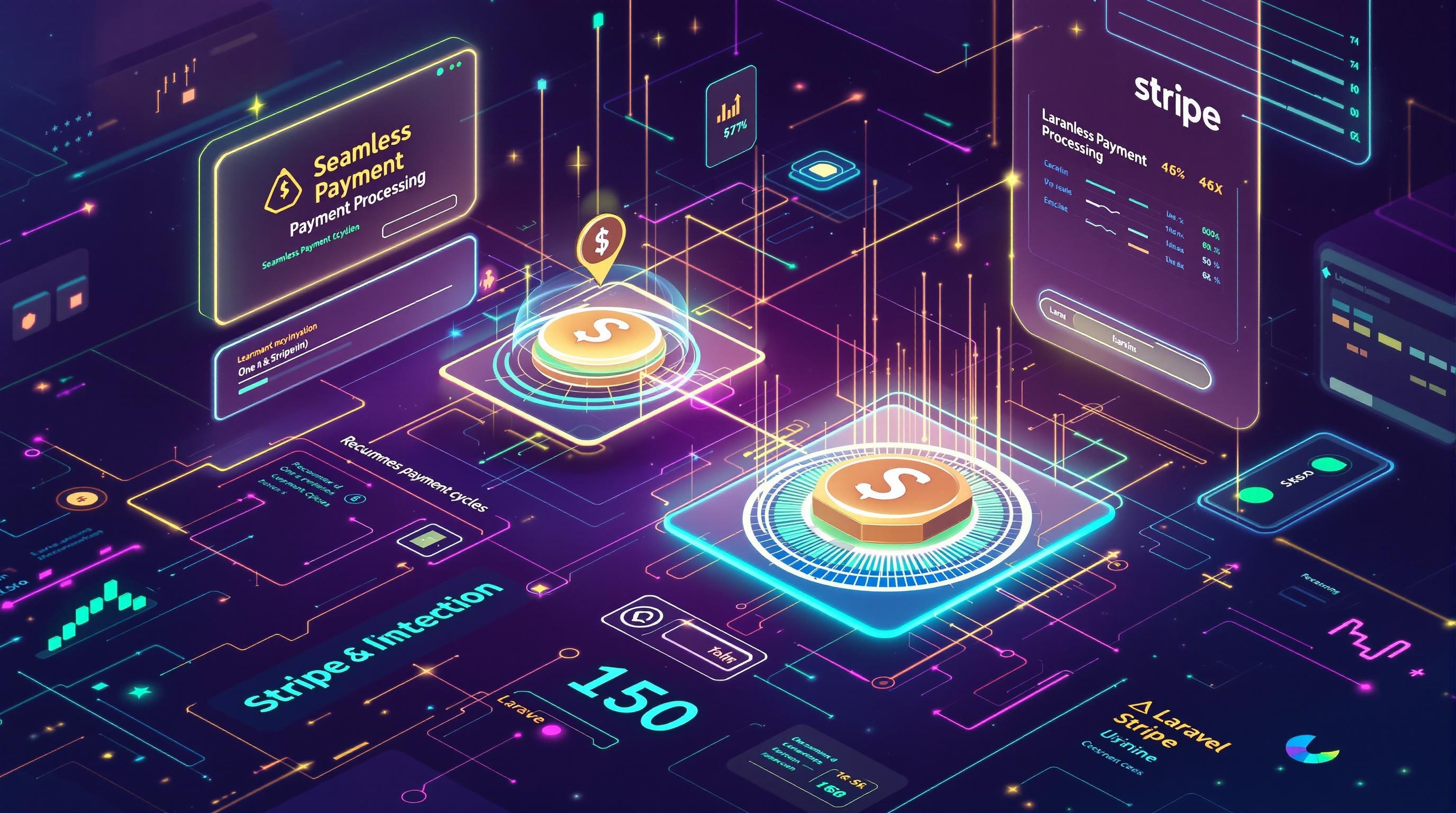
One-Time and Recurring Payments in Laravel Using Stripe
In the evolving landscape of digital commerce, having a robust payment processing system is crucial for businesses aiming to deliver seamless user experiences. One of the most effective ways to handle both one-time and recurring payments in a Laravel application is through Stripe—a versatile platform renowned for its ease of integration and powerful features.
Why Choose Stripe for Your Laravel Application?
Stripe is a developer-friendly platform that offers a comprehensive suite of tools for managing online payments. Its flexibility allows businesses to tailor solutions to meet specific needs, whether you’re managing one-time product sales or monthly subscription plans. Integrating Stripe with Laravel opens up opportunities to expand your payment options with minimal effort, thanks to Laravel's elegant syntax and robust framework.
Key Benefits of Using Stripe
- Global Reach: Stripe supports a wide range of currencies, allowing you to sell products and services worldwide.
- Secure Transactions: With built-in security features, Stripe ensures all transactions are protected, reducing the risk of fraud.
- Developer-Friendly: Comprehensive documentation and APIs make it easy for developers to customize their payment solutions.
- Scalable Solutions: Whether you are processing a few transactions a day or handling thousands, Stripe scales with your business.
Getting Started: Setting Up Stripe in Your Laravel Project
Before diving into code, you need to get your environment ready. Follow these steps to set up Stripe in your Laravel application.
Step 1: Install the Stripe Package
Begin by installing the Stripe PHP Library. This can be done using Composer, a dependency manager for PHP.
composer require stripe/stripe-php
This command will install the Stripe library, allowing you to use Stripe's API in your Laravel application.
Step 2: Configure Your Laravel Application
Next, configure your Stripe credentials in the Laravel application. Create a file named stripe.php in the config directory and fill it with your Stripe keys.
return [
'key' => env('STRIPE_KEY'),
'secret' => env('STRIPE_SECRET'),
];
Make sure these keys are defined in your .env file:
STRIPE_KEY=your_stripe_public_key
STRIPE_SECRET=your_stripe_secret_key
Handling One-Time Payments
To handle one-time payments, you need to create a payment form where users can input their payment information. Stripe Elements is a powerful UI component library that you can leverage to create responsive forms.
Step 3: Create a Payment Form
Create a new blade template that contains a simple form for collecting payment information using Stripe's form. Leverage Stripe.js to securely create payment tokens.
Example Blade Template
<form action="/charge" method="post" id="payment-form">
<div class="form-row">
<label for="card-element">
Credit or debit card
</label>
<div id="card-element"></div>
<div id="card-errors" role="alert"></div>
</div>
<button>Submit Payment</button>
</form>
Step 4: Processing the Payment
Once you have the payment form set up, you need to handle the form submission to process the payment and handle any errors. Insert your logic in the controller to communicate with Stripe's API and finalize the payment.
use Stripe\StripeClient;
class PaymentController extends Controller {
public function charge(Request $request) {
\Stripe\Stripe::setApiKey(config('stripe.secret'));
$amount = 5000; // Amount in cents
$payment_intent = \Stripe\PaymentIntent::create([
'amount' => $amount,
'currency' => 'usd',
'payment_method_types' => ['card'],
]);
return response()->json(['client_secret' => $payment_intent->client_secret]);
}
}
Recurring Payments: Setting Up Subscription Plans
Handling recurring payments requires setting up subscription plans that automatically bill your users on a scheduled basis. Here’s how you can manage subscriptions using Stripe.
Step 5: Define Your Subscription Plans
Start by defining your subscription plans in the Stripe dashboard. Provide details like price, billing cycle, and trial period if any.
Step 6: Integrate Subscription Logic in Laravel
After setting up your plans, integrate the subscription logic in your Laravel application. You will need to capture user details, their chosen subscription plan, and generate the subscription via Stripe's API.
use Stripe\Customer;
use Stripe\Subscription;
class SubscriptionController extends Controller {
public function subscribe(Request $request) {
$user = auth()->user();
\Stripe\Stripe::setApiKey(config('stripe.secret'));
$customer = Customer::create([
'email' => $user->email,
'source' => $request->stripeToken
]);
$subscription = Subscription::create([
'customer' => $customer->id,
'items' => [['price' => 'price_id']],
]);
return response()->json(['subscription' => $subscription]);
}
}
Managing Subscriptions
It's essential to have logic for managing and maintaining subscriptions. This includes upgrading or downgrading plans, attaching metadata to subscriptions, and handling webhooks for updates and notifications.
Step 7: Handling Webhooks for Real-Time Updates
Stripe provides webhooks which are crucial for receiving real-time updates about your subscriptions. Users can be notified about successful payments, subscription changes, or failures.
use App\Models\User;
class WebhookController extends Controller {
public function handleWebhook(Request $request) {
$event = $request->input('event');
switch ($event['type']) {
case 'invoice.payment_succeeded':
// Handle payment success
break;
case 'customer.subscription.updated':
// Handle subscription updates
break;
default:
// Unhandled event type
break;
}
}
}
Securing Your Payment Process
Security is paramount when handling payment information. Stripe automatically manages PCI compliance, but it's essential to follow best practices such as validating user input, handling unexpected errors gracefully, and safeguarding API keys.
Key Security Practices
- Validate Inputs: Ensure all data is validated before processing any payment.
- Handle Errors: Implement robust error handling and logging mechanisms.
- Store Credentials Securely: Use environment variables to store sensitive credentials securely.
Conclusion
By integrating Stripe with Laravel, you not only streamline your payment processes but also offer your customers a secure and efficient way to engage with your services. Whether handling one-time purchases or recurring subscription payments, Stripe’s robust API provides all the necessary tools to elevate your application's payment functionality.
Ready to elevate your Laravel application’s payment capabilities? Start with ZapKit, a Laravel-powered boilerplate designed to accelerate development, streamline payment integration, and bring your startup ideas to life faster.
For more tutorials and insights, check out our Dev Blog. Embrace the future of development with the right payment solutions today!