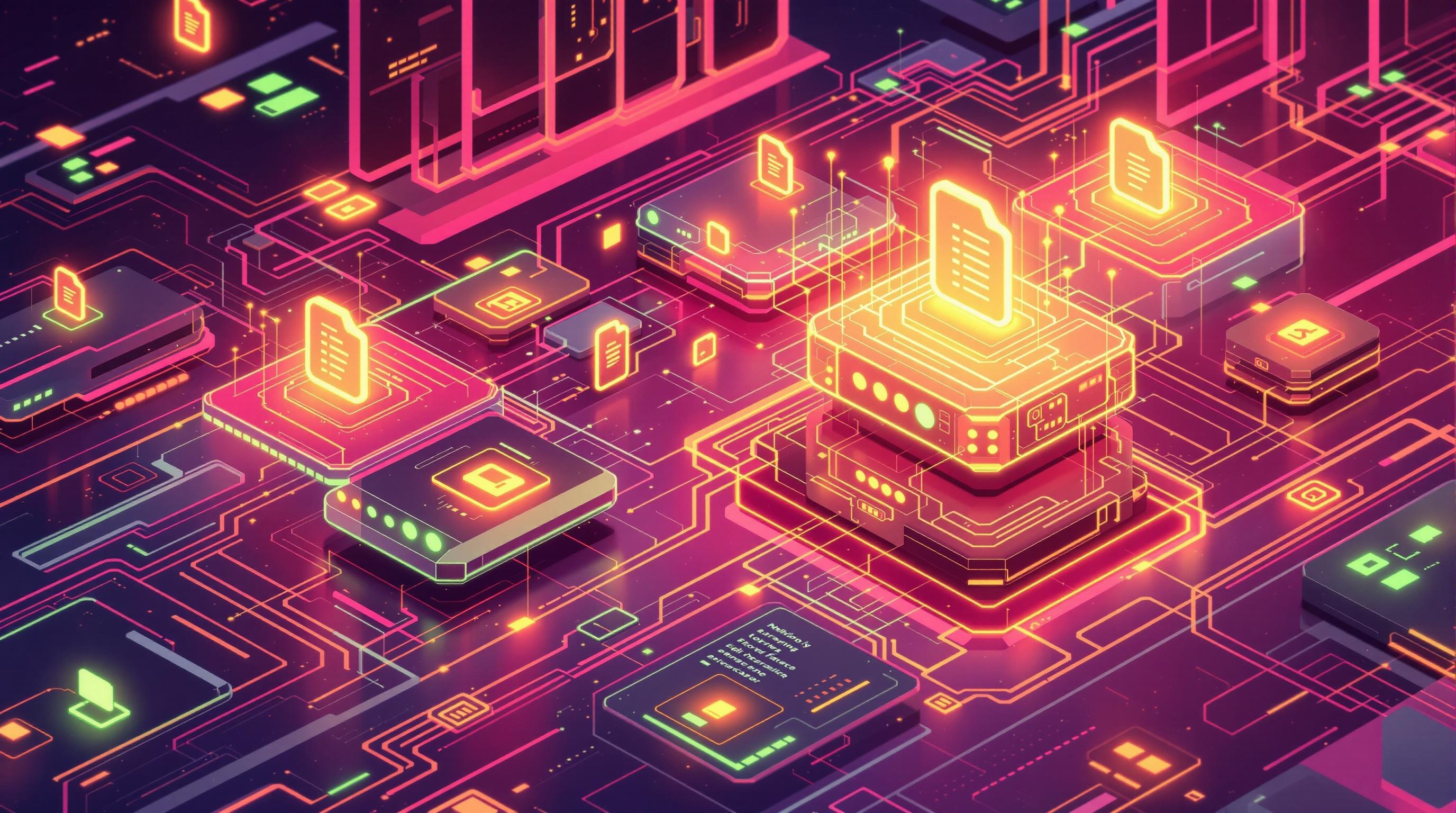
Mastering Laravel's Storage Facade for Efficient Media Management
When it comes to managing files, especially media in a web application, efficiency, and organization are paramount. Laravel, a PHP framework celebrated for its clean syntax and developer-friendly tools, offers a powerful component to facilitate file storage and retrieval: the Storage Facade. In this comprehensive guide, we will delve into Laravel's Storage Facade for effective media management, exploring file storage, retrieval options, and best practices to streamline your Laravel application.
Understanding Laravel's File Storage System
Laravel's file storage mechanism provides a unified API for interacting with local files and cloud storage services like Amazon S3, Google Cloud Storage, etc. This flexibility makes it an optimal choice for developers aiming to build scalable and robust applications capable of handling substantial media files.
Configuring the Filesystem
Out of the box, Laravel comes with support for multiple file systems. Configuration is made available via the filesystem.php
file located in the config
directory. It includes default settings for local and cloud drivers, allowing developers to specify their disk setup of choice.
Local Storage Configuration
Local disks store files within your application filesystem, allowing easy access and manipulation. To configure local storage, ensure your filesystem.php
is set to:
'default' => env('FILESYSTEM_DISK', 'local'),
'disks' => [
'local' => [
'driver' => 'local',
'root' => storage_path('app'),
],
Configuring Cloud Storage
For applications requiring cloud storage, Laravel is seamlessly integrated with services like AWS S3:
's3' => [
'driver' => 's3',
'key' => env('AWS_ACCESS_KEY_ID'),
'secret' => env('AWS_SECRET_ACCESS_KEY'),
'region' => env('AWS_DEFAULT_REGION'),
'bucket' => env('AWS_BUCKET'),
],
Leveraging Laravel’s Storage Facade
The Laravel Storage Facade provides an expressive and fluent interface for interacting with file systems. Here’s how you can manipulate your media files effectively:
Uploading Files
To upload a file using the Storage Facade, you can implement the put
method, which uploads file content to a specified path:
use Illuminate\Support\Facades\Storage;
$newFilePath = 'images/new-image.jpg';
$fileContents = file_get_contents('path/to/image.jpg');
Storage::put($newFilePath, $fileContents);
This method ensures your files are stored safely and can also handle streaming large files.
Retrieving Files
Retrieving stored files is equally straightforward. Using the get
method, you can fetch file contents:
$fileContents = Storage::get('path/to/file.jpg');
For large file retrieval, consider utilizing streaming capabilities to handle memory usage efficiently.
Generating File URLs
Laravel lets you generate URLs for your files stored in local or cloud storage using the url
method, which is perfect for dynamically displaying images:
$url = Storage::url('path/to/file.jpg');
Deleting Files
File deletions are managed with the delete
method:
Storage::delete('path/to/file.jpg');
This capability supports batch deletions, enabling quick purging of unwanted media assets.
Advanced File Storage Techniques
Larger applications often require advanced techniques to maintain speed and efficiency when handling media files. Here are some best practices:
Using Symbolic Links
Symbolic links can optimize file access speeds and manage public visibility through the php artisan storage:link
command, making files accessible from the web server without exposing the raw storage paths.
Versioning Files
For applications where files are frequently updated, implementing a file versioning system helps keep track of changes, ensuring users can access previous versions if necessary. This strategy involves naming conventions and database integration for metadata management.
Security and Performance Optimization
Ensuring the security and performance of your file storage system is crucial. Here are some strategies:
Implementing Permissions
Ensure your files have appropriate read/write permissions, and manage access using role-based authorization to protect sensitive media.
Caching Strategies
Cache frequently accessed files using Laravel’s caching mechanisms to reduce load times and server requests. This is particularly beneficial for media-heavy applications.
Compression and Optimization
Leverage tools to optimize image and video files, reducing their size without compromising quality to enhance load times and save storage space.
Integrating with Third-party Services
Larger projects might benefit from integration with third-party services for enhanced media handling. Platforms like Imgix, Cloudinary, or AWS MediaConvert offer advanced solutions for media transformation and delivery.
Conclusion
Managing media in your Laravel applications need not be an arduous task. By leveraging the Storage Facade and implementing the tips and techniques outlined in this guide, you can achieve seamless, scalable, and efficient media management. Whether working on a local setup or with cloud storage, Laravel offers the flexibility to meet your application's media management needs.
Interested in delving deeper into Laravel’s capabilities? Explore more tutorials and resources at ZapKit to enhance your development projects.
CTA: Ready to streamline your Laravel projects? Discover more about Laravel and accelerate your development with ZapKit's powerful tools and resources. Visit ZapKit.dev today!