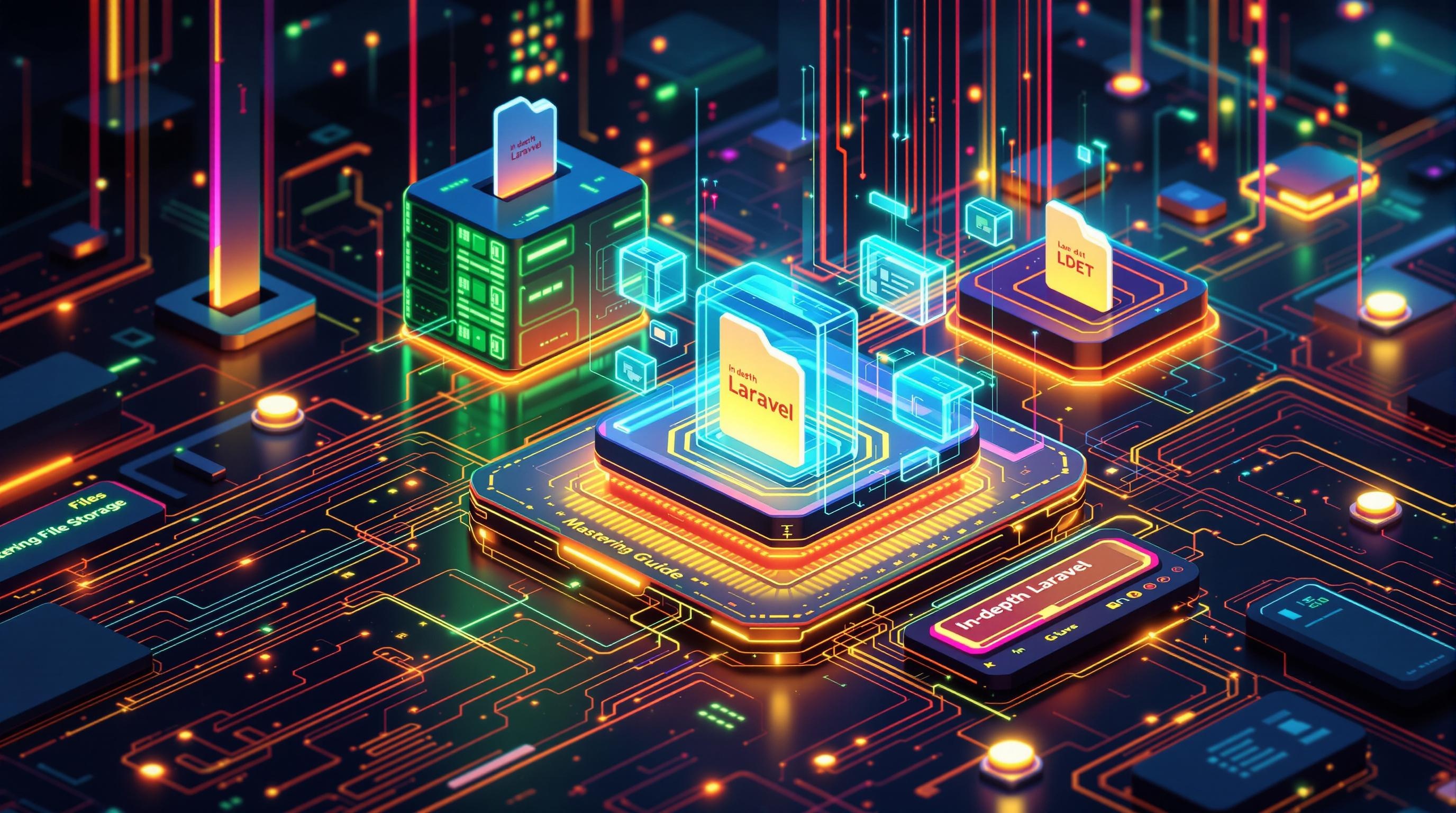
Mastering File Storage in Laravel: An In-Depth Guide
Laravel is one of the most popular PHP frameworks today, known for its elegance and simplicity. Among its many features, Laravel offers a robust file storage system that allows developers to handle files seamlessly. This guide will delve into the various aspects of file storage in Laravel, providing you with the insights needed to manage your file operations effectively.
Understanding Laravel's File Storage System
Laravel’s file storage is built on the Flysystem PHP package, providing a clean API for different filesystems. It supports local file storage, cloud-based stocks like Amazon S3, and many more. The abstraction within Laravel’s storage allows you to switch between file storage options quickly.
The Basics of Laravel's File Storage
Laravel's file storage is configured via the config/filesystems.php
file, where you can set up your default disk and specify the cloud disk options and root paths for various file systems. Here's a quick example:
return [
'default' => env('FILESYSTEM_DRIVER', 'local'),
'cloud' => env('FILESYSTEM_CLOUD', 's3'),
'disks' => [
'local' => [
'driver' => 'local',
'root' => storage_path('app'),
],
'public' => [
'driver' => 'local',
'root' => storage_path('app/public'),
'url' => env('APP_URL').'/storage',
'visibility' => 'public',
],
's3' => [
'driver' => 's3',
'key' => env('AWS_ACCESS_KEY_ID'),
'secret' => env('AWS_SECRET_ACCESS_KEY'),
'region' => env('AWS_DEFAULT_REGION'),
'bucket' => env('AWS_BUCKET'),
],
],
];
Accessing Files in Laravel
Once you've configured your file system, accessing files is straightforward. Laravel provides a Storage
facade to interact with file storage. Here’s a basic example of storing, retrieving, and deleting files:
- Storing a File:
This saves the uploaded file in the$path = $request->file('avatar')->store('avatars');
avatars
directory under the default disk. - Retrieving a File:
Retrieves the file named$file = Storage::get('file.jpg');
file.jpg
from storage. - Deleting a File:
Deletes the file namedStorage::delete('file.jpg');
file.jpg
from storage.
Advanced File Storage Techniques
As you get more comfortable with Laravel’s basic file storage operations, you'll find that the framework offers more advanced features to cater to complex needs.
Using Cloud Storage
Laravel makes it easy to work with cloud file storage solutions like Amazon S3. With configuration keys set in the .env
file, you can store and retrieve files from the cloud the same way you would with local storage:
$path = Storage::disk('s3')->put('avatars', $request->file('avatar'));
This line of code uploads a file directly to an S3 bucket under the avatars
directory.
File Visibility
One of the standout features of Laravel’s file storage is the ability to set file visibility. By default, files stored using the local driver are considered private, meaning they are only accessible by the application. However, you can easily set files to be publicly accessible:
Storage::disk('public')->put('file.jpg', $contents, 'public');
This makes the file accessible over the web, presuming you’ve set up a public disk correctly.
Custom Storage Paths
Laravel's flexibility extends to custom storage paths. You may want to save files into customized directories, which can be set directly in the code:
$path = Storage::put('custom/directory/file.txt', $contents);
Error Handling and Maintenance
Proper error handling and maintenance strategies are crucial for effective file storage management. Laravel makes this aspect straightforward by providing robust error reporting and handling tools.
Handling Exceptions
Laravel throws exceptions whenever file operations fail. It’s good practice to wrap your storage code in try-catch
blocks:
try {
Storage::disk('public')->put('file.jpg', $contents);
} catch (Exception $e) {
// Handle the exception
}
Logging and Monitoring
Implementing logging and monitoring solutions help track file storage operations. Laravel’s extensive logging capabilities allow you to log file operations such as files being created or deleted, and any errors encountered during these processes.
Security Practices
Security is paramount when dealing with file storage. Laravel offers several features to ensure that your file storage system is secure.
Encryption
For sensitive data, Laravel provides encryption tools to securely store files. You can encrypt files before storing them or use Laravel’s built-in encryption methods:
$encryptedContents = encrypt($contents);
Store the encrypted content securely within the Laravel framework.
Access Control
Using Laravel's roles and permissions system, you can control who has access to particular files. This is especially useful for applications that manage sensitive data, such as user-uploaded content.
Conclusion
Mastering file storage in Laravel is an essential part of building effective web applications. From basic storage techniques to advanced cloud implementations and security practices, Laravel’s robust file handling capabilities give developers the tools necessary for sophisticated file management. Whether you're just starting with Laravel or looking to deepen your understanding of its file storage features, mastering these essentials will serve you well.
For more insights and advanced Laravel techniques, explore the ZapKit platform and discover solutions that can take your Laravel projects to new heights. Start your free trial today!