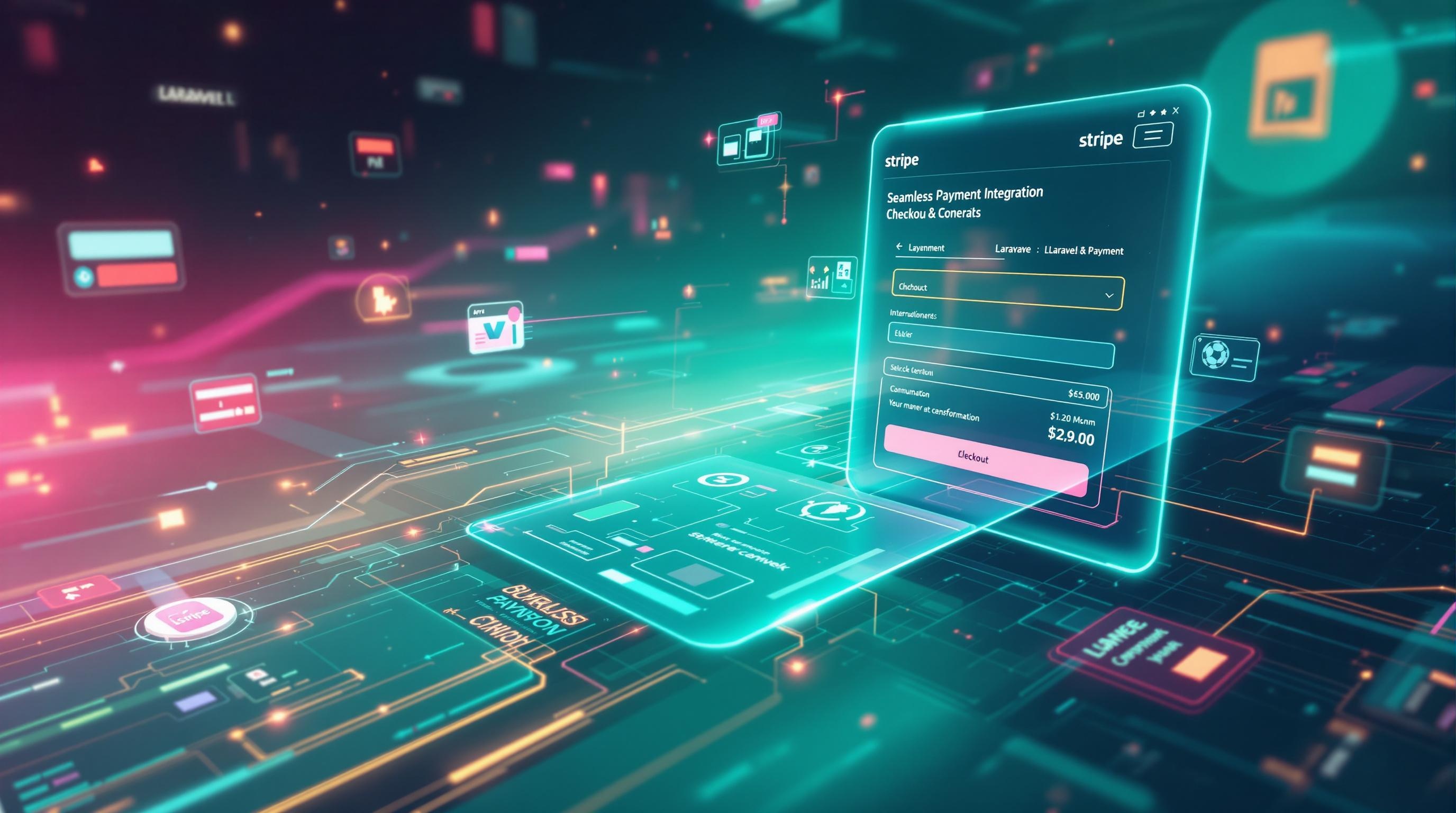
Integrating Stripe Payments into Your Laravel Application
In today's fast-paced digital economy, providing a secure and efficient payment processing system is crucial for any online business. Stripe, a leader in online payments, offers a robust solution for developers working with Laravel applications. In this comprehensive guide, we will walk you through the steps to integrate Stripe payments into your Laravel project, ensuring that your customers can enjoy a seamless transaction experience.
Why Choose Stripe for Your Laravel Application?
Stripe is renowned for its developer-friendly integrations and comprehensive feature set, making it an excellent choice for Laravel applications. Here's why Stripe is a popular choice:
- Ease of Use: Stripe's well-documented API and PHP library provide a seamless integration experience.
- Security: Stripe handles sensitive payment information securely, offering PCI compliance out of the box.
- Global Reach: With support for numerous currencies and payment methods, Stripe caters to a global audience.
- Scalability: Whether you're running a small business or a large enterprise, Stripe's infrastructure can scale to meet your needs.
Getting Started with Stripe and Laravel
Before diving into the integration process, ensure that you have a Laravel project set up and a Stripe account ready. Follow these preliminary steps:
- Set up a new Laravel project or navigate to your existing Laravel application directory.
- Create a Stripe account and obtain your API keys from the Stripe Dashboard.
- Install the Stripe PHP library using Composer with the command:
composer require stripe/stripe-php
Setting Up Stripe in Your Laravel Application
Now that you've prepared your environment, it's time to configure Stripe in your Laravel application. We'll start by updating your environment configuration:
Configure Environment Variables
Add your Stripe API keys to the .env
file for secure access:
STRIPE_KEY=your_stripe_public_key
STRIPE_SECRET=your_stripe_secret_key
Create a Service Provider
Next, create a service provider to bind the Stripe library to the Laravel application:
php artisan make:provider StripeServiceProvider
In the register
method of StripeServiceProvider.php
, add the following code:
use Stripe\Stripe;
public function register()
{
$this->app->singleton(Stripe::class, function () {
return new Stripe();
});
}
Route and Controller Setup
Let's define routes and create a controller to handle payment requests. In routes/web.php
, add:
Route::get('/payment', [PaymentController::class, 'showPaymentForm']);
Route::post('/process-payment', [PaymentController::class, 'processPayment']);
Create a controller using Artisan:
php artisan make:controller PaymentController
Building the Payment Form
Now, we need to build a frontend payment form that will send payment details to Stripe. Within your PaymentController
, implement:
public function showPaymentForm()
{
return view('payment-form');
}
Create a Blade View
Create a new Blade template file named payment-form.blade.php
in the resources/views
directory:
<form action="/process-payment" method="POST" id="payment-form">
@csrf
<input type="text" name="name" placeholder="Cardholder Name">
<input type="email" name="email" placeholder="Email">
<div id="card-element"></div>
<button type="submit">Pay</button>
</form>
<script src="https://js.stripe.com/v3/"></script>
<script>
const stripe = Stripe('{{ env('STRIPE_KEY') }}');
const elements = stripe.elements();
const cardElement = elements.create('card');
cardElement.mount('#card-element');
</script>
Processing Payments Securely
The final step is handling the payment on the server-side. Within your PaymentController
, add:
use Stripe\Charge;
public function processPayment(Request $request)
{
Stripe::setApiKey(env('STRIPE_SECRET'));
$charge = Charge::create([
'amount' => 999,
'currency' => 'usd',
'source' => $request->stripeToken,
'description' => 'Test payment from Laravel application',
]);
return back()->with('success', 'Payment Successful!');
}
Troubleshooting Common Issues
If you encounter issues such as payment failures or API errors, consider the following tips:
- Double-check your Stripe API keys in the
.env
file. - Ensure your server's date and time settings are correct for accurate signature validation.
- Consult the Stripe Documentation for specific error codes and solutions.
Enhancing the Payment Experience
Beyond the basic payment setup, you can enhance the user experience with Stripe's advanced features. Consider implementing:
- Webhooks: Use Stripe webhooks to handle events such as subscription updates and charge disputes.
- Stripe.js: Improve security by using Stripe Elements to tokenize card information client-side.
- Subscription Billing: Leverage Stripe's subscription API for recurring payment models.
Conclusion
Integrating Stripe payments within your Laravel application is a powerful way to ensure secure, efficient, and user-friendly payment processing. By following this guide, you've established a robust foundation for handling transactions reliably in your web application. For further customization and advanced features, explore the vast capabilities of Stripe and tailor the payment experience to your business needs.
Start integrating today with ZapKit and streamline your development with powerful boilerplates designed to launch your startup swiftly and efficiently.