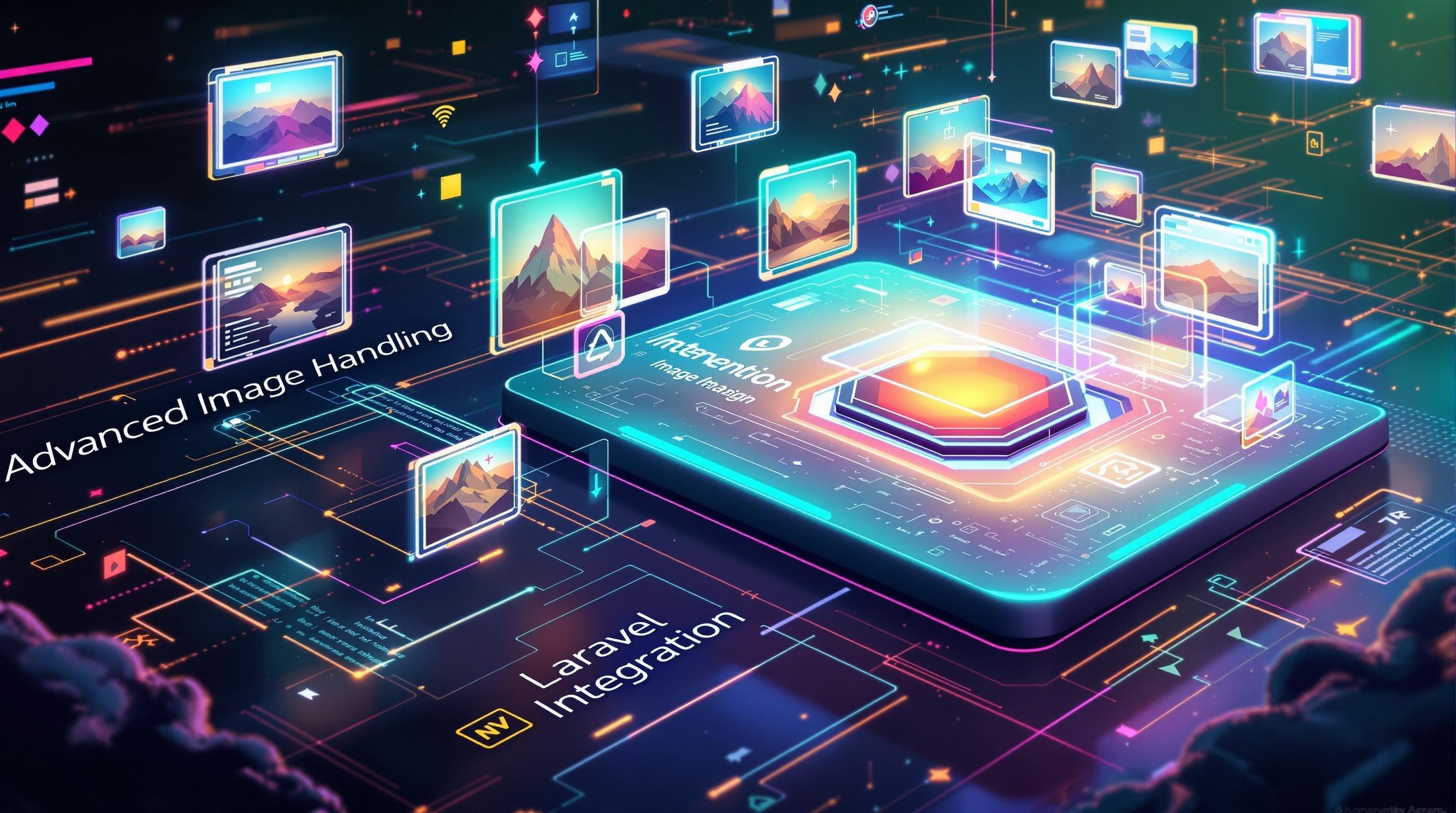
Image Upload and Processing in Laravel with Intervention Image
As web applications become more dynamic and visually appealing, developers increasingly need robust solutions for handling images. Laravel, a popular PHP framework, provides exceptional features for handling various web development tasks, including image upload and processing. By leveraging the power of the Intervention Image library, developers can easily manage images in Laravel, enhancing their applications' functionality and the user experience.
Understanding the Importance of Image Handling in Web Development
In modern web development, images are integral to content presentation and user interaction. They enhance website aesthetics, deliver essential information visually, and improve user engagement. However, handling images, especially in high-traffic scenarios, requires efficient methods to ensure fast load times and optimal performance.
Laravel, with its elegant syntax and robust community support, provides several tools and packages to facilitate seamless image handling. One such tool is Intervention Image, a PHP image handling and manipulation library designed to work effortlessly with Laravel applications.
Introducing Intervention Image
Intervention Image is a powerful open-source library that makes image manipulation easy and straightforward. With its Laravel integration, it provides methods for resizing, cropping, rotating, and optimizing images, making it an essential tool for developers seeking to enhance their application's image handling capabilities.
Setting Up Intervention Image in a Laravel Project
- Install the package via Composer: Start by adding Intervention Image to your Laravel project using Composer:
composer require intervention/image
- Register the service provider: After installation, register the service provider in your
config/app.php
file:
'providers' => [
// ...
Intervention\Image\ImageServiceProvider::class,
],
'aliases' => [
// ...
'Image' => Intervention\Image\Facades\Image::class,
],
Basic Image Upload in Laravel
Image upload in Laravel is made simple thanks to its HTTP request handling features. Here’s a basic overview of handling image uploads before processing:
- Create a form for file uploads: Add a file input in your HTML form:
<form action="/upload" method="post" enctype="multipart/form-data">
@csrf
<input type="file" name="image">
<button type="submit">Upload</button>
</form>
- Handle the request in a controller: Create a controller method to handle file uploads:
public function upload(Request $request)
{
$request->validate([
'image' => 'required|image|mimes:jpeg,png,jpg,gif|max:2048',
]);
$imageName = time().'.'.$request->image->extension();
$request->image->move(public_path('images'), $imageName);
return back()->with('success','Image Upload successful.');
}
Image Processing with Intervention Image
Once you've set up the basics for image uploads, you can now proceed to process these images using Intervention Image. Here’s how:
- Resizing the Image: Adjust image dimensions to suit your needs:
use Intervention\Image\Facades\Image;
public function resizeImage($filename)
{
$path = public_path('images/'.$filename);
$img = Image::make($path)->resize(300, 200);
$img->save($path);
return back()->with('success', 'Image resized successfully');
}
- Cropping the Image: Focus on essential parts of an image by cropping it:
public function cropImage($filename)
{
$path = public_path('images/'.$filename);
$img = Image::make($path)->crop(100, 100);
$img->save($path);
return back()->with('success', 'Image cropped successfully');
}
Optimizing Images for the Web
In addition to resizing and cropping, optimization is crucial for better performance. Intervention Image allows you to save images in a more optimized format:
$img->save($path, 75); // 75% of original quality
Reducing image quality can significantly cut file sizes, which is essential for faster load times and bandwidth savings without noticeably affecting visual quality.
Troubleshooting Common Issues
While working with image uploads and processing, you might encounter common issues such as permission errors, incorrect file paths, or unsupported image formats. Make sure to:
- Set the appropriate permissions for directories involved in storing uploaded files.
- Validate and sanitize file inputs to prevent unauthorized access.
- Keep your frameworks and libraries updated to avoid compatibility issues.
Conclusion
Handling images efficiently is a critical component of developing modern web applications. By using Laravel in conjunction with the Intervention Image library, developers can create highly functional, visually appealing applications with enhanced image handling capabilities. Whether you're working on a small project or a complex web application, these tools provide the flexibility and scalability you need.
Ready to take your Laravel projects to the next level with efficient image handling? Explore ZapKit for more tools and resources to accelerate your development process.
For further reading on Laravel and web development, check out our other articles: