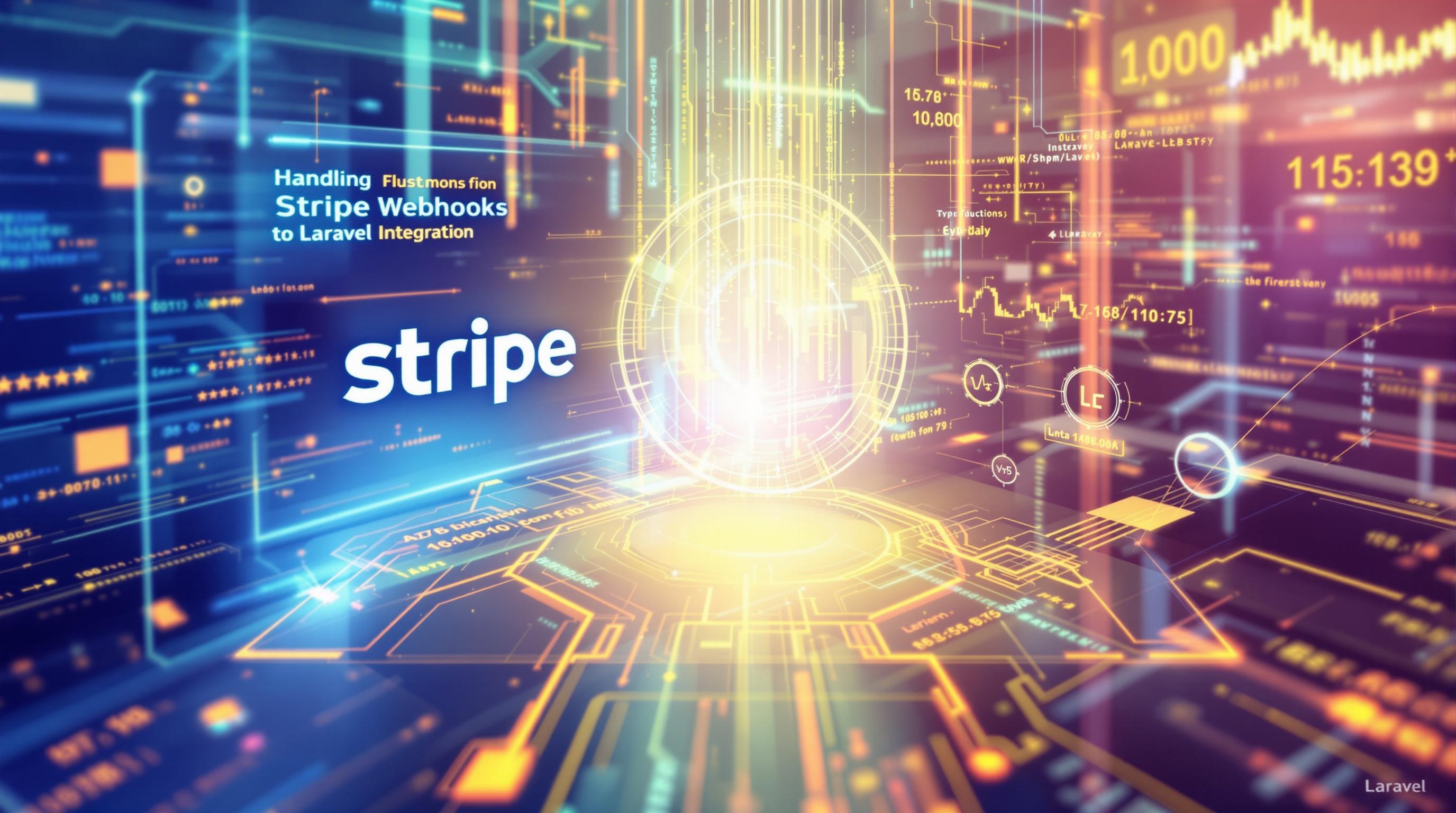
Handling Stripe Webhooks in Laravel: A Comprehensive Guide
Integrating payment solutions into web applications can be a challenging yet crucial task for developers. Stripe, a popular payment processing platform, provides a powerful way to handle financial transactions through webhooks. Webhooks are essential for real-time communication between your Laravel application and Stripe, allowing you to respond to events such as successful payments or subscription updates. This comprehensive guide will walk you through the process of handling Stripe webhooks in Laravel, covering setup, security, and troubleshooting.
Understanding Webhooks
Webhooks are automated messages sent from apps when something happens. Essentially, they provide a way for an app to send real-time data to other applications. In the context of Stripe and Laravel, webhooks allow Stripe to notify your Laravel application about events such as customer creation, payment updates, and subscription changes.
Why Use Stripe Webhooks?
- Real-time Updates: Webhooks ensure that your application gets real-time updates on various Stripe events.
- Automated Processes: It allows automating workflows, such as updating invoices or subscription details without continuous manual handling.
- Reliability: They reduce the risk of missed events since Stripe retries failed webhook delivery several times.
Setting Up Stripe Webhooks in Laravel
Step 1: Setting Up a Stripe Account
Before delving into webhook integration, ensure you have an active Stripe account. You will need your API keys, which you can find in your Stripe Dashboard under Developers > API keys.
Step 2: Create a Webhook Endpoint in Laravel
Create a new route in your Laravel application to handle incoming webhook requests. You can add this in your web.php
or api.php
file depending on your preference:
Route::post('/stripe/webhook', [StripeWebhookController::class, 'handle']);
Next, create a controller named StripeWebhookController
if you're following this file:
php artisan make:controller StripeWebhookController
Step 3: Handle Webhook Logic
In the StripeWebhookController
, add the `handle
` method to process the incoming webhook payload:
use Illuminate\Http\Request;
use Stripe\Stripe;
use Stripe\Event;
class StripeWebhookController extends Controller
{
public function handle(Request $request)
{
$payload = $request->getContent();
$sigHeader = $request->header('Stripe-Signature');
$webhookSecret = config('services.stripe.webhook_secret');
try {
$event = Event::constructFrom(
json_decode($payload, true),
$sigHeader,
$webhookSecret
);
// Handle the event
switch ($event->type) {
case 'payment_intent.succeeded':
$paymentIntent = $event->data->object; // contains a Stripe\PaymentIntent
break;
// Add more case statements to handle other event types
}
} catch (\UnexpectedValueException $e) {
// Invalid payload
response('Invalid payload', 400);
} catch (\Stripe\Exception\SignatureVerificationException $e) {
// Invalid signature
response('Invalid signature', 400);
}
return response('Webhook handled', 200);
}
}
Remember to replace `$webhookSecret` with your webhook secret from the Stripe dashboard under Developers > Webhooks. This helps verify that the incoming webhook is genuinely sent by Stripe.
Security Considerations
To ensure that your webhook endpoint is secure, consider the following measures:
- Authenticate Webhook Origin: Always validate that requests come from Stripe using the
Stripe-Signature
header. - HTTPS: Serve the webhook endpoint over HTTPS to encrypt traffic between your application and Stripe.
- Logging and Monitoring: Implement logging for webhook events to monitor your application's behavior and react to any suspicious activities promptly.
Troubleshooting Common Issues
While implementing Stripe webhooks, you may encounter certain challenges. Here are common issues and how to resolve them:
Issue 1: Invalid Signature Error
Ensure you are using the correct webhook secret. Verify that your server time is in sync as it can cause verification issues.
Issue 2: Missing Events
Check your event types in the Stripe dashboard. Ensure you're subscribed to all necessary events that your application needs to handle.
Issue 3: Unexpected Errors
Make use of Stripe's extensive logging and dashboard to investigate any unusual behavior or errors affecting your webhooks.
Additional Resources and References
- Stripe Webhooks Documentation - The official documentation provides detailed insights into webhooks usage, configuration, and best practices.
- Laravel HTTP Routing - Learn more about setting up HTTP routes in Laravel applications.
- ZapKit - Explore how ZapKit can help you accelerate your Laravel development.
Conclusion
Effectively handling webhooks is a vital component in building robust, responsive SaaS applications. With the integration of Stripe webhooks into your Laravel project, you can automate your application to respond to various payment and user events in real-time. This guide covers the critical aspects of setting up and securing Stripe webhooks in Laravel, offering you a comprehensive approach to managing your online payment processes efficiently. Start enhancing your Laravel applications today by implementing these techniques, and explore more features with tools like ZapKit.
Happy developing!
Call to Action: Ready to take your Laravel applications to the next level? Visit ZapKit to discover powerful developer tools to accelerate your development journey.