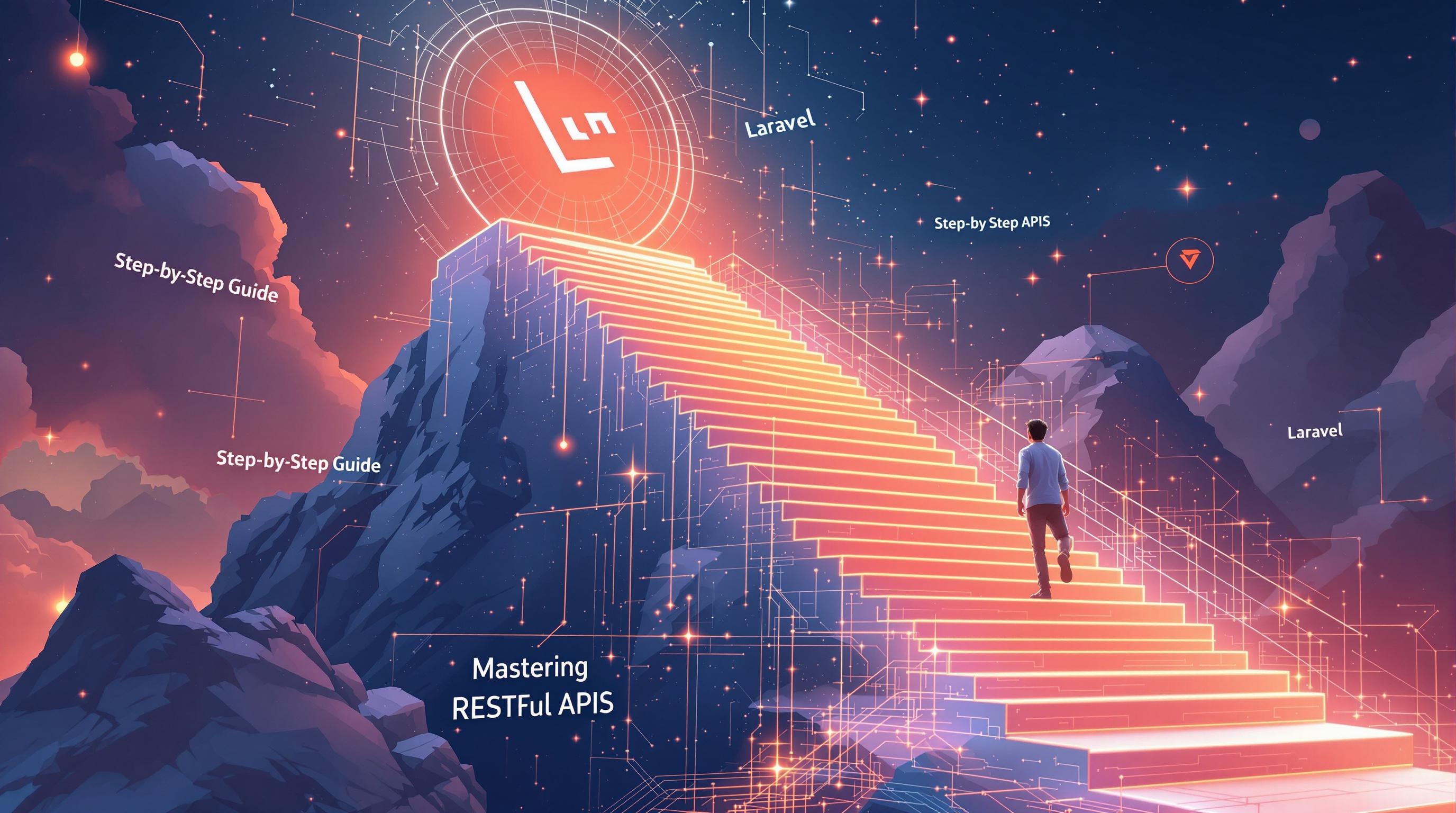
Building RESTful APIs with Laravel: A Step-by-Step Guide
In today’s digital era, APIs have become the core component of modern web and mobile application development. Among various API types, RESTful APIs stand out due to their simplicity, scalability, and stateless nature. Laravel, a robust PHP framework, has been a preferred choice for developers looking to create scalable API solutions. In this guide, we'll walk through building a RESTful API using Laravel, offering a clear path from setup to deployment.
Why Choose Laravel for Building APIs?
Before diving into the process, it’s essential to understand why Laravel is a popular choice for API development:
- Eloquent ORM: Laravel's powerful ORM simplifies database interaction.
- Built-in Authentication: Laravel provides built-in methods and functionality to handle authentication, essential for securing APIs.
- Scalability: Laravel applications are highly scalable, suiting small to enterprise-level applications.
- Robust Community: A thriving community contributes to and supports Laravel, ensuring you have access to resources and help when needed.
Setting Up the Development Environment
Before you start building your API, ensure your development environment is correctly set up. You need PHP, Composer, and Laravel installed. Follow these steps to get started:
1. Install Composer
Composer is a dependency manager for PHP, essential for managing Laravel's dependencies. You can download Composer from the official website.
2. Install Laravel
Once Composer is installed, you can install Laravel globally with the following command:
composer global require laravel/installer
3. Create a New Laravel Project
Use the Laravel installer to create a new project:
laravel new restful-api
This command sets up a new Laravel project named "restful-api" in a directory of the same name.
Designing the API Structure
Before writing code, it’s critical to design your API. The design should outline your resources, endpoints, HTTP methods, and response formats. Tools like Swagger can help in this phase.
Identify Resources
Determine what resources your API will manage. For example, if you're building a blog API, core resources might include Posts, Users, and Comments.
Building the API
With the design in hand, let's start building the API. We'll walk through creating endpoints for a simple blog API as an example.
1. Define Routes
Laravel routes are defined in the routes/api.php
file. You specify the endpoint URIs, the HTTP method, and which controller action it should call. For example:
Route::get('/posts', 'PostController@index');
Route::post('/posts', 'PostController@store');
Route::get('/posts/{id}', 'PostController@show');
Route::put('/posts/{id}', 'PostController@update');
Route::delete('/posts/{id}', 'PostController@destroy');
2. Create Controllers
Controllers handle incoming requests and perform actions on the data. Generate a controller using Artisan, Laravel’s command-line interface:
php artisan make:controller PostController --resource
The --resource
flag generates boilerplate CRUD methods: index
, store
, show
, update
, and destroy
.
3. Manage Data with Eloquent
Laravel’s Eloquent allows for fluent interaction with the database. Define a model and connect it to your database table:
php artisan make:model Post -m
The -m
flag also generates a migration file, allowing you to define the database schema.
4. Run Migrations
Migrations are Laravel’s way of version controlling database changes. Define your table structure in the migration files, then run them using:
php artisan migrate
This command applies the migrations, creating database tables.
Implementing Authentication
Securing your API is crucial. Laravel simplifies API authentication with Passport, Laravel’s OAuth2 server implementation. Here’s a basic setup:
1. Install Passport
composer require laravel/passport
2. Configure Passport
After installation, use the following Artisan command to create the encryption keys necessary for secure access:
php artisan passport:install
Add the HasApiTokens
trait to your User model:
use Laravel\Passport\HasApiTokens;
class User extends Authenticatable
{
use HasApiTokens, Notifiable;
}
Testing the API
Testing ensures your API is reliable and bug-free. Use Laravel’s HTTP tests to automate testing:
php artisan test
This command runs all tests defined in the tests/Feature
directory. Use assertions to verify response structures and data integrity.
Deploying Your API
Once your API is built and tested, the next step is deployment. Laravel Forge or Vapor are excellent services tailored for Laravel applications, offering seamless deployments. Ensure environment variables are properly configured on the server and use a tool like Forge for streamlining deployment and server management.
Conclusion
Building RESTful APIs with Laravel provides a strong foundation for developing scalable and secure applications. With its powerful tools and extensive community support, Laravel simplifies tasks from routing to authentication. Whether you’re creating a simple application or a complex enterprise system, mastering Laravel API development opens numerous opportunities.
Ready to build your project with Laravel? Visit ZapKit.dev for innovative solutions and tools to accelerate your development process.
Call to Action: Explore our other blogs to expand your development skills and master Laravel with ease!