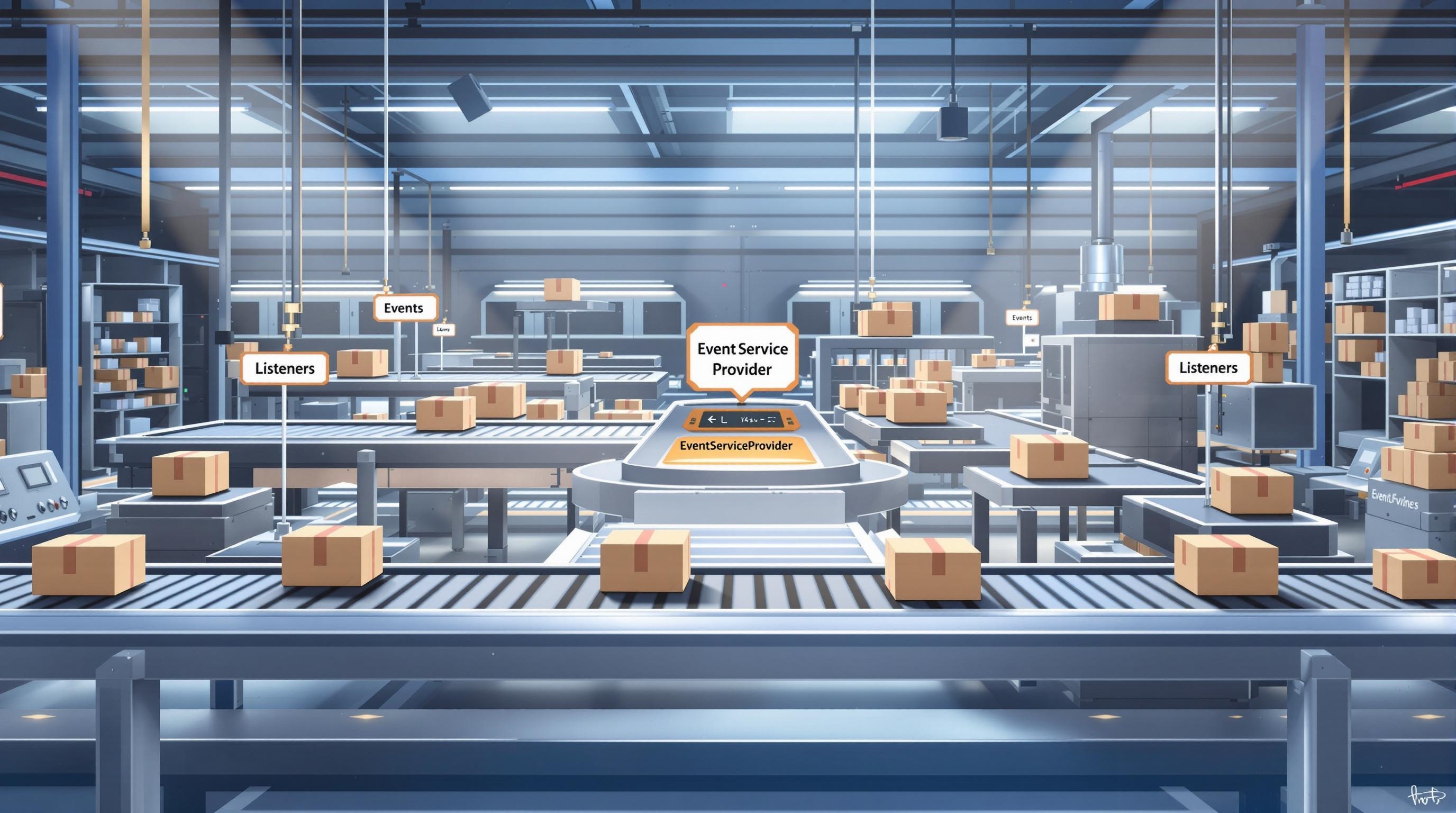
Building Decoupled Applications Using Laravel's Event System
In the ever-evolving landscape of software development, creating applications that are both flexible and scalable is paramount. One of the architectural approaches that supports such objectives is decoupling components within your application. Laravel, a popular PHP framework, offers a powerful toolset to achieve this through its event system. Decoupling through events not only enhances scalability but also leads to cleaner code and improved maintainability.
Understanding Decoupled Architecture
Before diving into Laravel's event system, it's crucial to comprehend what decoupled architecture means. Simply put, it refers to designing systems where components are independent of each other, allowing them to interact without being tightly bound. This separation of concerns makes it easier to change one part of the application without affecting others, fostering a more robust and adaptable application structure.
Benefits of Decoupling
- Scalability: Decoupled systems can scale efficiently, as they facilitate the addition or modification of components independently.
- Maintainability: Such architecture simplifies updates and maintenance, reducing the risk of unforeseen bugs.
- Flexibility: New features can be integrated seamlessly, enhancing the application's capability.
- Reusability: Components can be reused across different projects, saving time and resources.
Laravel's Event System: A Brief Overview
Laravel provides a robust event system that allows developers to inject decoupled behavior into their applications easily. At its core, the event system is designed to listen for and respond to various events across the application.
Core Components of the Event System
- Events: These are classes that represent something that has occurred within your application. Events can carry data, which event listeners can use to perform further actions.
- Listeners: These are classes that respond to the events. They contain logic that should execute when a specific event occurs.
- Event Service Provider: This registers the events and listeners within the application.
Steps to Implement Event-Driven Architecture in Laravel
Implementing event-driven architecture in Laravel involves several key steps. Let's explore these with practical guidance.
Step 1: Define Events
Begin by defining the events that will trigger specific actions in your application. Use the Artisan command to create an event:
php artisan make:event UserRegistered
This command generates an event class, like UserRegistered, which can carry information about the user who registered.
Step 2: Define Listeners
Listeners handle the logic that should be executed when an associated event occurs. Use the following Artisan command to generate a listener:
php artisan make:listener SendWelcomeEmail
The listener, such as SendWelcomeEmail, contains the code necessary to send an email when a user registers.
Step 3: Register Events and Listeners
Next, register the events and listeners within the EventServiceProvider. Open app/Providers/EventServiceProvider.php and map your events to listeners:
protected $listen = [ 'App\Events\UserRegistered' => [ 'App\Listeners\SendWelcomeEmail', ], ];
Step 4: Trigger Events
Events can be triggered from anywhere within your application. For instance, after a user registers, you might dispatch the UserRegistered event:
event(new UserRegistered($user));
Step 5: Handling Multiple Listeners
Laravel allows multiple listeners for a single event. This feature is particularly useful for executing a sequence of actions in response to a single occurrence, such as logging activity, queuing related tasks, or updating a dashboard.
Best Practices for Using Laravel's Event System
While using the event system can bring significant benefits, adhering to best practices ensures you maximize its potential without introducing complexity.
Use Events for Milestone Achievements
Events should signify significant actions or milestones within your application. Avoid creating events for minor operations, which can lead to unnecessary complexity.
Avoid Overusing Listeners
While it's tempting to create listeners for numerous tasks, it's wise to focus on core functionalities and maintain simplicity. Leverage queues for time-consuming listener operations to keep response times optimal.
Keep Event Data Simple
Ensure that the data carried by events is minimal and relevant. Passing large amounts of data can increase memory usage and decrease performance.
Real-World Applications of Event-Driven Architecture
Event-driven architecture is employed in a variety of contexts. From e-commerce platforms to social networking applications, the possibilities are vast.
E-commerce Platforms
In an online store, events such as adding items to a cart, placing an order, or changing an order's status are pivotal. Listeners reacting to these events can handle inventory management, notify logistics, or update user interfaces accordingly.
Social Networking Applications
Social networks rely heavily on event-driven systems. Events like new post creation, likes, shares, and comments trigger subsequent actions such as notifications and feed updates.
Exploring Advanced Event Features in Laravel
Lesser-known but powerful features of Laravel's event system can further enhance application development.
Event Broadcasting
Broadcasting allows server-side events to be sent to connected clients in real-time, providing updates like notifications or live comments. This is crucial for applications where real-time interaction is key.
Middleware for Events
Although not as common, you can attach middleware to events that process additional logic, authentication, or validation before a listener executes.
Conclusion
Building decoupled applications using Laravel's event system offers a streamlined approach to managing complex interactions within your app. By efficiently leveraging events and listeners, developers can enhance an application's scalability, maintainability, and overall architecture. As you explore Laravel further, consider incorporating event-driven architecture into your development practice to unlock new potentials for flexibility and adaptability.
Start experimenting with Laravel's event system now, and explore the unlimited possibilities it brings to software architecture. For more insights on Laravel and startup-friendly development strategies, visit ZapKit today!