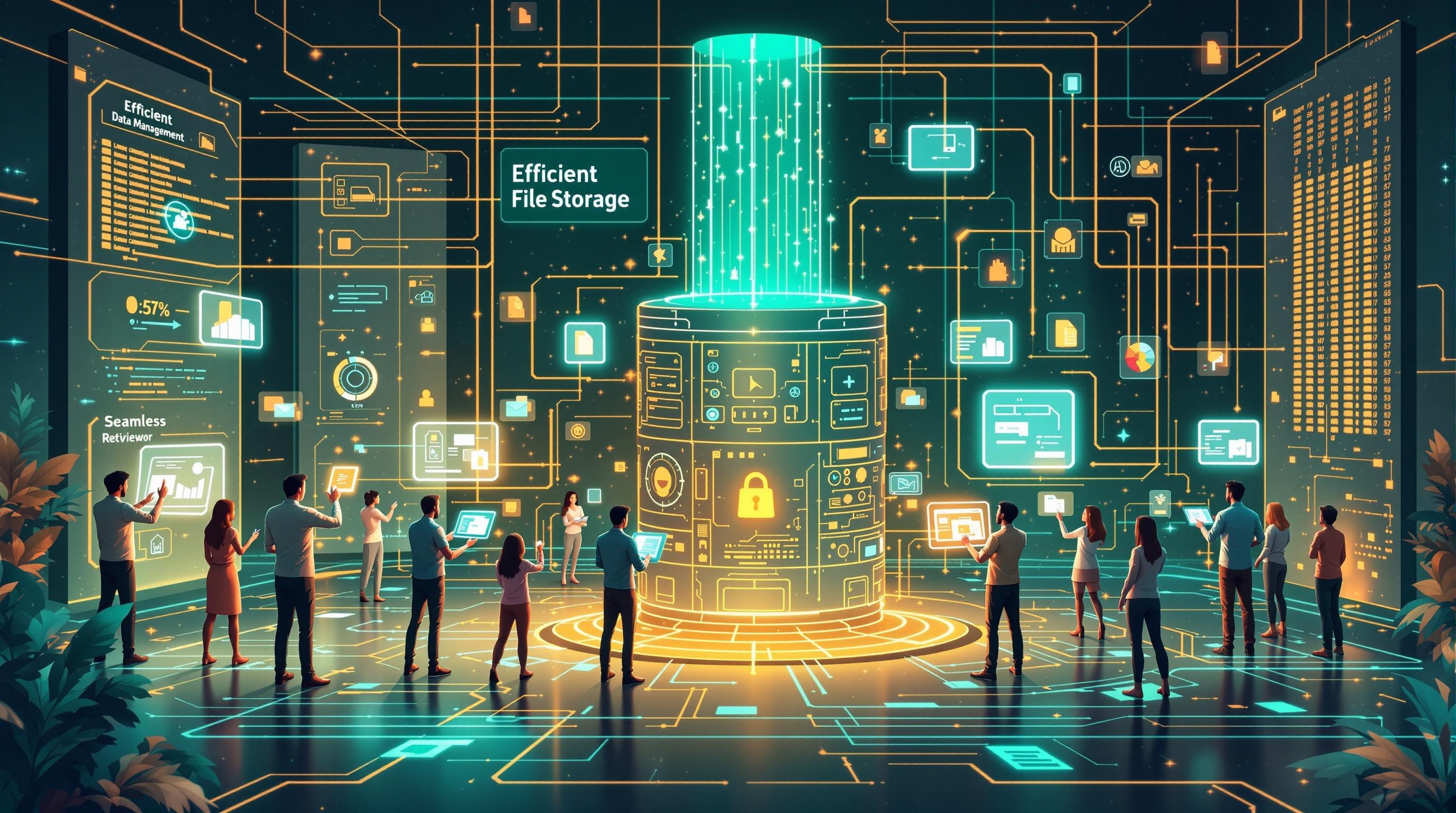
Best Practices for Storing and Retrieving Files in Laravel
Laravel, a popular PHP framework, is renowned for its elegant syntax and ease of use, making it a favorite among developers for web application development. One of the core features of Laravel is its robust file storage system, which facilitates storing and retrieving files with ease. In this comprehensive guide, we will explore the best practices for managing file storage and retrieval in Laravel.
Understanding Laravel's File Storage System
Before diving into best practices, it’s essential to understand how Laravel’s file storage system works. Laravel provides a powerful filesystem abstraction through the Filesystem
facade. This abstraction presents a unified interface to interact with different storage backends, such as local disk, Amazon S3, and FTP.
Configuring Your File Storage
Laravel uses a configuration file named filesystems.php
located in the config
directory. This file is where different disk configurations are defined. These disks can be local, public, or cloud-based. Setting up these configurations correctly is critical for efficient file operations.
Example configuration for local and S3 disks:
'disks' => [
'local' => [
'driver' => 'local',
'root' => storage_path('app'),
],
's3' => [
'driver' => 's3',
'key' => env('AWS_ACCESS_KEY_ID'),
'secret' => env('AWS_SECRET_ACCESS_KEY'),
'region' => env('AWS_DEFAULT_REGION'),
'bucket' => env('AWS_BUCKET'),
],
],
Storing Files in Laravel
Choosing the Right Storage Driver
The first step in any storage operation is selecting the appropriate storage driver. Laravel offers support for local, public, Amazon S3, and more. Your choice should depend on the project requirements, budget, and desired performance.
Using the Storage Facade
Laravel’s Storage
facade provides a simple, expressive interface for interacting with storage disks. Here’s how you can store a file:
use Illuminate\Support\Facades\Storage;
// Store a file in the 'local' disk
Storage::disk('local')->put('file.txt', 'Contents');
// Store an uploaded file
$request->file('photo')->store('photos', 'public');
It is crucial to ensure files are stored in the correct directories and are assigned proper access permissions. This practice helps maintain security and organization within your storage system.
Validating Uploaded Files
Before storing files, validate them to safeguard your application from malicious uploads. Laravel makes this easy with the built-in validation:
request()->validate([
'photo' => 'required|mimes:jpg,png,jpeg,gif|max:2048',
]);
This validation rule ensures that only images are uploaded and restricts the maximum file size for security and performance.
Retrieving Files in Laravel
Efficient File Retrieval
When retrieving files in Laravel, efficiency is key. Use the Storage
facade to access files:
// Get file contents
$content = Storage::disk('local')->get('file.txt');
// Download a file
return Storage::download('file.txt');
If working with large files, consider streaming them to reduce memory usage. Laravel supports streaming using the storage:link
command, particularly useful for serving public files:
php artisan storage:link
Handling Missing Files Gracefully
Always anticipate potential errors such as missing files. Laravel provides methods to check for file existence and handle such cases gracefully:
if (Storage::disk('local')->exists('file.txt')) {
$file = Storage::disk('local')->get('file.txt');
} else {
abort(404);
}
Security Best Practices
Protect Sensitive Files
Security should be a top priority when managing file storage and retrieval. Always ensure private files are not publicly accessible by storing them outside of the web root. Files that need restricted access should use signed URLs or authentication checks.
Encrypt Files for Added Security
Laravel supports file encryption, an effective method to protect sensitive data:
use Illuminate\Support\Facades\Storage;
// Encrypt the file
Storage::put('file.txt', encrypt($content));
// Decrypt the file
$content = decrypt(Storage::get('file.txt'));
Performance Optimization Tips
Use Caching Wisely
Caching frequently accessed files can improve your application's performance. Consider using Laravel’s cache mechanism for files that don’t change often. Redis or Memcached are supported backends that work seamlessly with Laravel.
Choose the Right Storage Strategy
Depending on the application’s requirements, a hybrid approach can be optimal, mixing cloud storage with local caching. This strategy enhances performance while keeping costs manageable.
Conclusion
Mastering file storage and retrieval in Laravel involves understanding its file system, following best security practices, and optimizing for performance. By implementing these best practices, you can ensure your Laravel application is efficient, secure, and scalable. Enhance your Laravel projects with these strategies, and explore further capabilities of the framework to harness its full potential.
Ready to build amazing applications with Laravel? Check out ZapKit for more resources and tools to boost your development process!